Elastic Off-canvas Navigation with jQuery and CSS3
File Size: | 2.41 KB |
---|---|
Views Total: | 6654 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
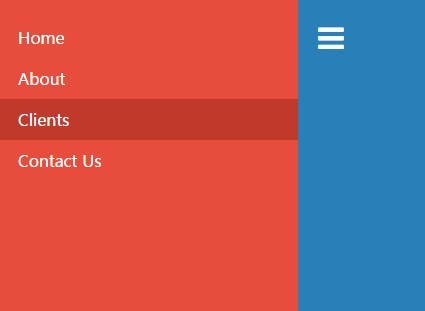
A modern mobile app style navigation solution that makes uses of jQuery and CSS3 to create an off-canvas sidebar push menu with a fancy elastic effect.
How to use it:
1. Include the Font Awesome in the head section (Optional. Just for menu toggle icon).
<link rel="stylesheet" href="//netdna.bootstrapcdn.com/font-awesome/4.3.0/css/font-awesome.min.css">
2. Create a trigger button to toggle the off-canvas push menu.
<div class="button"> <div class="fa fa-bars"></div> </div>
3. Create a nav list that contains menu links pointing to the content sections of your web page.
<div class="menu"> <nav> <ul> <li> <a href="home_is_visible">Home</a> </li> <li> <a href="aboutus_is_visible">About</a> </li> <li> <a href="clients_is_visible">Clients</a> </li> <li> <a href="contactus_is_visible">Contact Us</a> </li> </ul> </nav> </div>
4. Create sections of content as follow.
<div class="content home"> <h1>Home</h1> <p>...</p> </div> <div class="content aboutus"> <h1>About Us</h1> <p>...</p> </div> <div class="content clients"> <h1>Clients</h1> <p>...</p> </div> <div class="content contactus"> <h1>Contact Us</h1> <p>...</p> </div>
5. The general CSS styles.
* { box-sizing: border-box; } html { overflow-x: hidden; } body { color: #fff; font-family: 'roboto'; position: relative; background: #E74C3C; -webkit-transform: translateX(0); -ms-transform: translateX(0); transform: translateX(0); -webkit-transition: -webkit-transform 800ms cubic-bezier(0.68, -0.55, 0.265, 1.55); transition: transform 800ms cubic-bezier(0.68, -0.55, 0.265, 1.55); margin: 0; padding: 0; } h1 { font-size: 60px; } p { margin-bottom: 100px; line-height: 2.4; } ul { margin: 0; padding: 20px 0; list-style: none; } li { margin: 0; padding: 0; } a { text-decoration: none; display: block; padding: 10px 20px; padding-left: 120px; } a:hover { background: #C0392B; }
6. The core CSS/CSS3 styles for the off-canvas push menu.
.button { position: absolute; top: 10px; left: 10px; z-index: 10000; color: white; padding: 10px; font-size: 30px; cursor: pointer; } .button .fa { -webkit-transition: color 1000ms cubic-bezier(0.68, -0.55, 0.265, 1.55); transition: color 1000ms cubic-bezier(0.68, -0.55, 0.265, 1.55); -webkit-transition-delay: .75s; transition-delay: .75s; } .menu { position: absolute; top: 0; left: 0; bottom: 0; width: 400px; padding-left: 0; -webkit-transform: translateX(-150%); -ms-transform: translateX(-150%); transform: translateX(-150%); -webkit-transition: -webkit-transform 500ms cubic-bezier(0.68, -0.55, 0.265, 1.55); transition: transform 500ms cubic-bezier(0.68, -0.55, 0.265, 1.55); -webkit-transition-delay: .2s; transition-delay: .2s; } .content { position: absolute; margin: 0 auto; padding: 30px 20%; font-size: 18px; -webkit-transform: translateX(100%); -ms-transform: translateX(100%); transform: translateX(100%); -webkit-transition: color 1s ease 1s, background 0.5s ease 1s, -webkit-transform 1000ms cubic-bezier(0.68, -0.55, 0.265, 1.55) 0.5s; transition: color 1s ease 1s, background 0.5s ease 1s, transform 1000ms cubic-bezier(0.68, -0.55, 0.265, 1.55) 0.5s; } .home_is_visible .home, .aboutus_is_visible .aboutus, .clients_is_visible .clients, .contactus_is_visible .contactus { -webkit-transform: translateX(0); -ms-transform: translateX(0); transform: translateX(0); } .home_is_visible .home, .aboutus_is_visible .aboutus, .clients_is_visible .clients, .contactus_is_visible .contactus { z-index: 5000; } .home_is_visible .home { color: white; background: #2980B9; } .home_is_visible .button .fa { color: white; } .home_is_visible a { color: white; } .aboutus_is_visible .aboutus { color: black; background: salmon; } .aboutus_is_visible .button .fa { color: black; } .aboutus_is_visible a { color: black; } .clients_is_visible .clients { color: white; background: darkgoldenrod; } .clients_is_visible .button .fa { color: white; } .clients_is_visible a { color: white; } .contactus_is_visible .contactus { color: black; background: sandybrown; } .contactus_is_visible .button .fa { color: black; } .contactus_is_visible a { color: black; } body.nav_is_visible { -webkit-transform: translateX(300px); -ms-transform: translateX(300px); transform: translateX(300px); } body.nav_is_visible .menu { -webkit-transform: translateX(-100%); -ms-transform: translateX(-100%); transform: translateX(-100%); }
7. Add the following javascript snippet after jQuery library to enable the off-canvas push menu.
$('body').addClass('home_is_visible'); $('.button').on('click', function () { $('body').toggleClass('nav_is_visible'); }); function removeClasses() { $('.menu ul li').each(function () { var link = $(this).find('a').attr('href'); $('body').removeClass(link); }); } $('.menu a').on('click', function (e) { e.preventDefault(); removeClasses(); var link = $(this).attr('href'); $('body').addClass(link); $('body').removeClass('nav_is_visible'); });
This awesome jQuery plugin is developed by tylerfowle. For more Advanced Usages, please check the demo page or visit the official website.