Dynamic Autocomplete Tag Input Plugin For jQuery - Tokenize2
File Size: | 38.6 KB |
---|---|
Views Total: | 39352 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
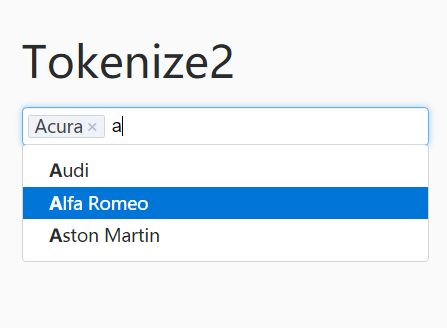
Tokenize2 is an easy-to-use jQuery plugin for tokenizing text input that allows to select multiple tags from a drop down list populated with suggestions defined in the select
element and/or an external JSON file (via AJAX requests).
Compatible with Twitter Bootstrap 3/4 framework.
How to use it:
1. Load the Tokenize2 and Bootstrap's style sheets in the head
section of the webpage.
<link rel="stylesheet" href="bootstrap.min.css"> <link rel="stylesheet" href="tokenize2.css">
2. Load jQuery library and the Tokenize2 plugin's JavaScript file at the bottom of the webpage.
<script src="//code.jquery.com/jquery.min.js"></script> <script src="tokenize2.js"></script>
3. Load jQuery UI library to make the tags sortable (OPTIONAL).
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.min.js"></script>
4. Suppose you have a multiple
select list as this:
<select class="demo" multiple> <option value="1">Acura</option> <option value="2">Audi</option> <option value="3">BMW</option> <option value="4">Cadillac</option> <option value="5">Chevrolet</option> <option value="6">Ferrari</option> <option value="7">Ford</option> <option value="8">Honda</option> <option value="9">Lexus</option> <option value="10">Mercedes-Benz</option> </select>
5. Call the function to convert the select element into a tag input.
$('.demo').tokenize2();
6. If you'd like to load auto suggestions from an external JSON file via AJAX requests.
$('.demo-1').tokenize2({ dataSource: 'remote.php' });
// JSON [ {"text": "Afghanistan", "value": "AF"}, {"text": "Åland Islands", "value": "AX"}, {"text": "Albania", "value": "AL"}, {"text": "Algeria", "value": "DZ"}, {"text": "American Samoa", "value": "AS"}, {"text": "Andorra", "value": "AD"}, {"text": "Angola", "value": "AO"} ]
7. All configuration options with default values.
$('.demo').tokenize2({ // max number of tags tokensMaxItems: 0, // allow you to create custom tokens tokensAllowCustom: false, // max items in the dropdown dropdownMaxItems: 10, // allow you to choose if the first item is automatically selected on search dropdownSelectFirstItem: true, // minimum/maximum of characters required to start searching searchMinLength: 0, searchMaxLength: 0, // specify if Tokenize2 will search from the begining of a string searchFromStart: true, // choose if you want your search highlighted in the result dropdown searchHighlight: true, // custom delimiter delimiter: ',', // display no results message displayNoResultsMessage: false, noResultsMessageText: 'No results mached "%s"', // custom delimiter delimiter: ',', // data source dataSource: 'select', // waiting time between each search debounce: 0, // custom placeholder text placeholder: false, // enable sortable // requires jQuery UI sortable: false, // tabIndex tabIndex: 0, // allows empty values allowEmptyValues: false, // z-inde zIndexMargin: 500 });
8. Event handlers.
$('.demo').on('tokenize:load', function(e){ // do something }); $('.demo').on('tokenize:clear', function(e){ // do something }); $('.demo').on('tokenize:remap', function(e){ // do something }); $('.demo').on('tokenize:focus', function(e){ // do something }); $('.demo').on('tokenize:deselect', function(e){ // do something }); $('.demo').on('tokenize:search', function(e, searchValue){ // do something }); $('.demo').on('tokenize:paste', function(e){ // do something }); $('.demo').on('tokenize:clear', function(e){ // do something }); $('.demo').on('tokenize:dropdown:up', function(e){ // do something }); $('.demo').on('tokenize:dropdown:down', function(e){ // do something }); $('.demo').on('tokenize:dropdown:clear', function(e){ // do something }); $('.demo').on('tokenize:dropdown:show', function(e){ // do something }); $('.demo').on('tokenize:dropdown:hide', function(e){ // do something }); $('.demo').on('tokenize:dropdown:fill', function(e, items){ // items: [array of object] }); $('.demo').on('tokenize:dropdown:itemAdd', function(e, value){ // do something }); $('.demo').on('tokenize:keypress', function(e, routedEvent){ // do something }); $('.demo').on('tokenize:keydown', function(e, routedEvent){ // do something }); $('.demo').on('tokenize:keyup', function(e, routedEvent){ // do something }); $('.demo').on('tokenize:tokens:reorder', function(e){ // do something }); $('.demo').on('tokenize:tokens:add', function(e, value, text, force){ // value The token value // text The token display text // force Must be true if tokensAllowCustom is false }); $('.demo').on('tokenize:tokens:added', function(e, value, text){ // value The token value // text The token display text }); $('.demo').on('tokenize:tokens:remove', function(e, value){ // do something });
Changelog:
v1.3.5 (2021-06-02)
- Support of CTRL+A to mark all tokens for delete (input search must be empty)
- Fix escaping functions
v1.3.3 (2020-11-30)
- Add new option dropdownSelectFirstItem
- Add missing trim function
v1.3.2 (2020-01-27)
- Fix dropdown fill with remote source
2019-12-27
- Added events.
2019-12-26
- Add option to limit length of tokens on manually adding
2017-05-06
- Fix shift tab
2017-05-05
- Add auto z-index for dropdown
This awesome jQuery plugin is developed by dragonofmercy. For more Advanced Usages, please check the demo page or visit the official website.