User-friendly Autocomplete Plugin For Text Input - AutoSuggestList
File Size: | 23.7 KB |
---|---|
Views Total: | 2411 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
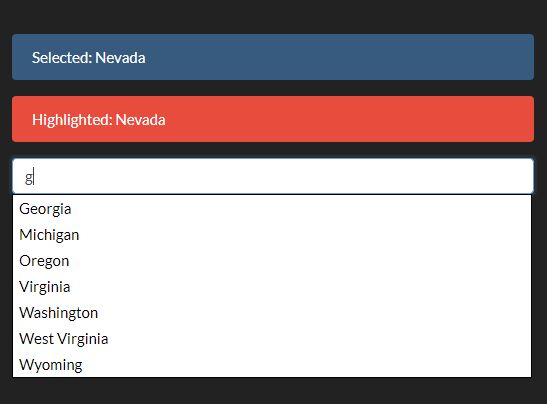
AutoSuggestList is a jQuery & jQuery UI based autocomplete plugin that adds an auto-suggestion box to the normal input field while typing.
The auto-suggestion box dynamically renders suggestive search results from JS strings or JSON objects with any properties.
How to use it:
1. Load the needed jQuery and jQuery UI libraries in the html file.
<script src="https://code.jquery.com/jquery-3.3.1.min.js" integrity="sha384-tsQFqpEReu7ZLhBV2VZlAu7zcOV+rXbYlF2cqB8txI/8aZajjp4Bqd+V6D5IgvKT" crossorigin="anonymous"> </script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.min.js"></script>
2. Download and load the latest version of AutoSuggestList plugin after jQuery.
<script src="/path/to/auto-suggest-list.js"></script>
3. Define the suggestions in the JavaScript as follows:
var myData = ['string1', 'string2', 'string3']; // or var myData =[ {id: 1, name: 'name1', prop: 'customProp1'}, {id: 2, name: 'name2', prop: 'customProp2'}, {id: 3, name: 'name3', prop: 'customProp3'} ];
4. Attach the AutoSuggestList plugin to your text field. Done.
<input type="text" id="example">
$('#example').autoSuggestList({ list: myData });
5. Apply your own CSS styles to the suggestion box.
$('#example').autoSuggestList({ divCss: {}, divClasses: '', ulCss: {}, ulClasses: '', liCss: {}, liClasses: '', highlightCss: {}, highlightClasses: '' });
6. Configuration options with default values.
$('#example').autoSuggestList({ // property name to display displayProperty: null, // decide whether each item matches the search text searchMatchCondition: null, // what value should be put in the textbox when an item is selected textBoxFormatter: null, // the minimum amount of characters to trigger the search minSearchChars: 0, // search delay in ms searchDelay: 0, // is case sensitive? matchCase: false, // shows full suggestion list on focus showFullListOnFocus: true, // auto highlights the first option autoSelectFirst: false, // auto highlight the item when there is only one in the suggestion list autoSelectOnly: true, // delay in ms of item highlight scroll when holding arrow keys (0 for the browser's default behavior) // useful if you want to make the scroll speed with arrow keys very fast or very slow arrowHoldScrollInterval: 0 });
7. Event handlers available.
$('#example').autoSuggestList({ itemSelected: function(event, item) { // when selected }, itemHighlighted: function(event, item) { // when highlighted }, itemMouseenter: function(event, item) { // before itemHighlighted }, itemMouseleave: function(event, item) { // on mouse leave } }); // or $('#example').on('asl-itemselected', function(event, item) {}); $('#example').on('asl-itemhighlighted', function(event, item) {}); $('#example').on('asl-itemmouseenter', function(event, item) {}); $('#example').on('asl-itemmouseleave', function(event, item) {});
This awesome jQuery plugin is developed by sayelr. For more Advanced Usages, please check the demo page or visit the official website.