Cookie-enabled Exam Wizard Plugin With jQuery - examWizard.js
File Size: | 22.6 KB |
---|---|
Views Total: | 4376 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
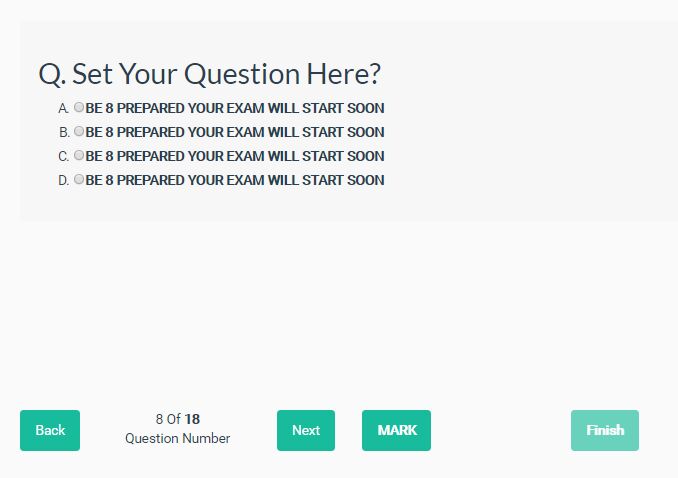
A full-featured and cookie-enabled jQuery exam wizard plugin that helps you create online mock exams, tests, homework, surveys with ease.
Key features:
- Supports as many questions.
- Stores the answers in the cookies and restore them when needed.
- Next/Prev buttons.
- Allows you to set questions using any form fields: checkbox, radio button, select box, text field, etc.
- Displays answers in a quick access menu.
- Allows to mark questions.
- Callback functions.
How to use it:
1. Load the minified version of the jQuery examWizard.js plugin after jQuery.
<script src="https://code.jquery.com/jquery-1.12.4.min.js" integrity="sha384-nvAa0+6Qg9clwYCGGPpDQLVpLNn0fRaROjHqs13t4Ggj3Ez50XnGQqc/r8MhnRDZ" crossorigin="anonymous"> </script> <script src="js/examwizard.min.js"></script>
2. Create an HTML form for the exam wizard and insert your own questions to the form as follows:
<form id="myForm"> <div class="question" data-question="1"> <input type="radio" data-alternatetype="radio" name="fieldName[0]" data-alternateName="answer[0]" data-alternateValue="A" value="1" id="answer-0-1"/> <label for="answer-0-1" class="answer-text"><span></span>BE 1 PREPARED YOUR EXAM WILL START SOON</label> <input type="radio" data-alternatetype="radio" name="fieldName[0]" data-alternateName="answer[0]" data-alternateValue="B" value="2" id="answer-0-2"/><label for="answer-0-2" class="answer-text"><span></span>BE 1 PREPARED YOUR EXAM WILL START SOON</label> <input type="radio" data-alternatetype="radio" name="fieldName[0]" data-alternateName="answer[0]" data-alternateValue="C" value="3" id="answer-0-3"/> <label for="answer-0-3" class="answer-text"><span></span>BE 1 PREPARED YOUR EXAM WILL START SOON</label> ... </div> <div class="question" data-question="2"> More questions here </div> ... <input type="hidden" value="1" id="currentQuestionNumber" name="currentQuestionNumber" /> <input type="hidden" value="18" id="totalOfQuestion" name="totalOfQuestion" /> <input type="hidden" value="[]" id="markedQuestion" name="markedQuestions" /> </form>
3. Create a quick access panel to display question numbers and anwsers.
<div id="quick-access-section"> <table> <thead class="question-response-header"> <tr> <th class="text-center">Question</th> <th class="text-center">Response</th> </tr> </thead> <tbody> <tr class="question-response-rows" data-question="1"> <td>1</td> <td class="question-response-rows-value">-</td> </tr> <tr class="question-response-rows" data-question="2"> <td>2</td> <td class="question-response-rows-value">-</td> </tr> ... </tbody> </table> <div> <a href="javascript:void(0)" id="quick-access-prev">< Back</a> <span id="quick-access-info"></span> <a href="javascript:void(0)" id="quick-access-next">Next ></a> </div> </div>
4. Create the footer containing navigation & pagination controls for the exam wizard.
<div class="exams-footer"> <div class="back-to-prev-question-wrapper text-center"> <a href="javascript:void(0);" id="back-to-prev-question"> Back </a> </div> <div class="footer-question-number-wrapper"> <span id="current-question-number-label">1</span> <span>Of <b>18</b></span> Question Number </div> <div class="go-to-next-question-wrapper"> <a href="javascript:void(0);" id="go-to-next-question" class="btn btn-success"> Next </a> </div> <div> <div class="mark-unmark-wrapper" data-question="1"> <a href="javascript:void(0);" class="mark-question" data-question="1"> <b>MARK</b> </a> <a href="javascript:void(0);" class="hidden unmark-question" data-question="1"> <b>UNMARK</b> </a> </div> <div class="mark-unmark-wrapper hidden" data-question="2"> <a href="javascript:void(0);" class="mark-question" data-question="2"> <b>MARK</b> </a> <a href="javascript:void(0);" class="hidden unmark-question" data-question="2"> <b>UNMARK</b> </a> </div> ... </div> <div class="footer-finish-question-wrapper"> <a href="javascript:void(0);" id="finishExams"> <b>Finish</b> </a> </div> </div>
5. Initialize the exam wizard and done.
var examWizard = $.fn.examWizard();
6. Possible plugin options to customize the exam wizard.
var examWizard = $.fn.examWizard({ currentQuestionSelector:'#currentQuestionNumber', totalOfQuestionSelector:'#totalOfQuestion', formSelector: '#examwizard-question', currentQuestionLabel: '#current-question-number-label', alternateNameAttr: 'data-alternateName', alternateValueAttr: 'data-alternateValue', alternateTypeAttr: 'data-alternateType', quickAccessOption: { quickAccessSection: '#quick-access-section', enableAccessSection: true, quickAccessPagerItem: 'Full', quickAccessInfoSelector:'#quick-access-info', quickAccessPrevSelector:'#quick-access-prev', quickAccessNextSelector:'#quick-access-next', quickAccessInfoSeperator:'/', quickAccessRow: '.question-response-rows', quickAccessRowValue: '.question-response-rows-value', quickAccessDefaultRowVal:'-', quickAccessRowValSeparator: ', ', nextCallBack :function(){}, prevCallBack :function(){}, }, nextOption: { nextSelector: '#go-to-next-question', allowadNext: true, callBack: function(){}, breakNext: function(){return false;}, }, prevOption: { prevSelector: '#back-to-prev-question', allowadPrev: true, allowadPrevOnQNum: 2, callBack: function(){}, breakPrev: function(){return false;}, }, finishOption: { enableAndDisableFinshBtn:true, enableFinishButtonAtQNum:'onLastQuestion', finishBtnSelector: '#finishExams', enableModal: false, finishModalTarget: '#finishExamsModal', finishModalAnswerd: '.finishExams-total-answerd', finishModalMarked: '.finishExams-total-marked', finishModalRemaining: '.finishExams-total-remaining', callBack: function(){} }, markOption: { markSelector: '.mark-question', unmarkSelector: '.unmark-question', markedLinkSelector: '.marked-link', markedQuestionsSelector:'#markedQuestion', markedLabel: 'Marked', markUnmarkWrapper: '.mark-unmark-wrapper', enableMarked: true, markCallBack: function(){}, unMarkCallBack: function(){}, }, cookiesOption: { enableCookie: false, cookieKey: '', expires: 1*24*60*60*1000 // 1 day } });
This awesome jQuery plugin is developed by aneeshikmat. For more Advanced Usages, please check the demo page or visit the official website.