Simple jQuery Form Wizard with Input Validation - Simple Form
File Size: | 8.41 KB |
---|---|
Views Total: | 27830 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
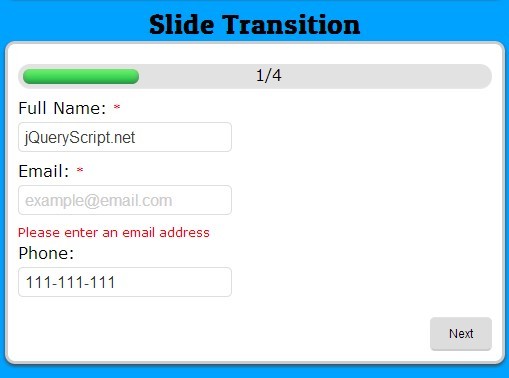
Simple Form is a jQuery plugin that turns a form into a multi-step wizard with support for form validation and progress indication.
You might also like:
- Powerful jQuery Form Wizard Plugin - formwizard
- jQuery Form Wizard Plugin - Smart Wizard
- jQuery Multi-Step Form Wizard Plugin
How to use it:
1. Include the needed jQuery library and jQuery validation plugin in your document.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script src="http://ajax.aspnetcdn.com/ajax/jquery.validate/1.12.0/jquery.validate.min.js"></script>
2. Include the jQuery simple form plugin after jQuery library.
<link rel="stylesheet" href="simpleform.css"> <script src="simpleform.min.js"></script>
3. Create a standard form that will be separated into several steps based on fieldset
<form class="testform" id="testform" method="post"> <fieldset class="personal-data"> <label for="name">Full Name: <span class="required">*</span></label> <input type="text" id="name" name="name" placeholder="John Smith" /> <label for="email-address">Email: <span class="required">*</span></label> <input type="email" name="email" id="email" placeholder="[email protected]" /> <label for="phone">Phone:</label> <input type="tel" name="phone" id="phone" placeholder="000-000-000" /> </fieldset> <fieldset class="address-data-inputs"> <label for="house-id">Building Number:</label> <input type="text" id="house-id" name="house-id" placeholder="1" /> <label for="street">Street: <span class="required">*</span></label> <input type="text" id="street" name="street" placeholder="The Street" /> <label for="town">Town:</label> <input type="text" id="town" name="town" placeholder="A Town"/> <label for="county">County:</label> <input type="text" id="county" name="county" placeholder="San Luis Obispo"/> <label for="postal-code">Postal Code:</label> <input type="text" id="postal-code" name="postal-code" placeholder="93401" /> </fieldset> <fieldset class="message-details"> <label for="comments">Comments:</label> <textarea name="comments" id="comments"></textarea> </fieldset> <div class="clear"></div> </form>
4. Call the plugin on the form with optional settings.
$(".testform").simpleform({ speed : 500, // animation speed transition : 'fade', // fade or slide progressBar : true, // display a progress indicator showProgressText : true, // display progress text validate: true // enable form validation });
5. Custom the rules and messages for the form validator. Check out the jQuery validation plugin's official website for more details.
Obj.validate({ rules: { email: { required: true, email: true }, name: { required: true }, street: { required: true } }, messages: { email: { required: "Please enter an email address", email: "Not a valid email address" }, name: { required: "Please enter your name" }, street: { required: "Please enter street name" } } });
6. All the default options.
next : 'Next', previous : 'Previous', submit : 'Submit', transition : 'fade', speed : 500, validate : false, progressBar : true, showProgressText : true,
This awesome jQuery plugin is developed by RobGraham. For more Advanced Usages, please check the demo page or visit the official website.