Simple jQuery & jQuery UI Autocomplete Plugin For Textareas
File Size: | 3.64 KB |
---|---|
Views Total: | 15079 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
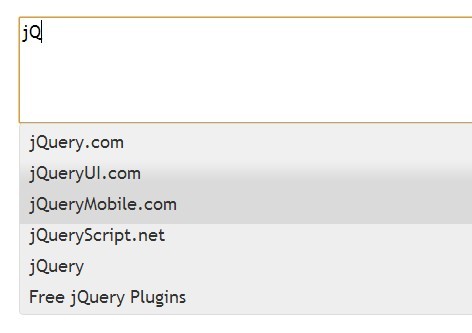
There're a lot of jQuery based autocomplete plugin for input fields out there. Today I'm going to introduce a jQuery & jQuery UI script that allows you to create autocomplete/autosuggest boxes for textareas. All this does is allows you to query each word being typed into a textarea against a list of words in an array.
How to use it:
1. Load the latest jQuery javascript library in the page.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.0/jquery.min.js"></script>
2. Load the jQuery UI's javascript and CSS files in the page.
<link rel="stylesheet" type="text/css" href="http://code.jquery.com/ui/1.10.4/themes/ui-lightness/jquery-ui.css"/> <script src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.10.4/jquery-ui.min.js"></script>
3. Create a standard textarea on the page.
<textarea id="demo"></textarea>
4. The javascript.
<script> $(function() { var availableTags = ["jQuery.com", "jQueryUI.com", "jQueryMobile.com", "jQueryScript.net", "jQuery", "Free jQuery Plugins"]; // array of autocomplete words var minWordLength = 2; function split(val) { return val.split(' '); } function extractLast(term) { return split(term).pop(); } $("#demo") // jQuery Selector // don't navigate away from the field on tab when selecting an item .bind("keydown", function(event) { if (event.keyCode === $.ui.keyCode.TAB && $(this).data("ui-autocomplete").menu.active) { event.preventDefault(); } }).autocomplete({ minLength: minWordLength, source: function(request, response) { // delegate back to autocomplete, but extract the last term var term = extractLast(request.term); if(term.length >= minWordLength){ response($.ui.autocomplete.filter( availableTags, term )); } }, focus: function() { // prevent value inserted on focus return false; }, select: function(event, ui) { var terms = split(this.value); // remove the current input terms.pop(); // add the selected item terms.push(ui.item.value); // add placeholder to get the comma-and-space at the end terms.push(""); this.value = terms.join(" "); return false; } }); }); </script>
This awesome jQuery plugin is developed by johncarmichael. For more Advanced Usages, please check the demo page or visit the official website.