Fully Featured Form Serializer In jQuery - serializeJSON
File Size: | 148 KB |
---|---|
Views Total: | 1056 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
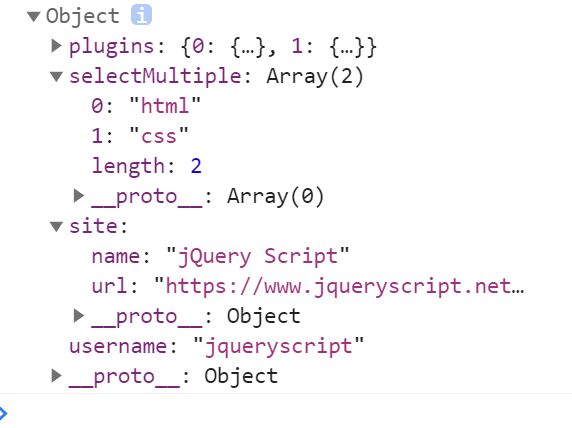
serializeJSON is a robust jQuery based form serializer that makes it easier to serialize your form data into a JavaScript object containing key/value pairs for further use.
Key Features:
- Easy to use.
- Supports nested attributes and arrays.
- Supports almost all form fields like input, select, checkbox, ...
- Supports custom data types: string, boolean, number, array, object, ...
See Also:
How to use it:
1. Insert the JavaScript file jquery.serializejson.min.js
after loading jQuery library and we're ready to go.
<script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/jquery.serializejson.js"></script>
2. Call the function on your HTML form to serialize the form data into a JavaScript object.
<form> <!-- Basic --> <input type="text" name="username" value="jqueryscript" /> <!-- Nested Attributes --> <input type="text" name="site[name]" value="jQuery Script" /> <input type="url" name="site[url]" value="https://www.jqueryscript.net/" /> <!-- Nested Arrays --> <input type="hidden" name="plugins[0][popular]" value="0" /> <input type="checkbox" name="plugins[0][popular]" value="1" checked /> <textarea name="plugins[1][name]"></textarea> <!-- Select --> <select multiple name="selectMultiple[]"> <option value="html" selected>HTML</option> <option value="css" selected>CSS</option> <option value="js">JavaScript</option> </select> </form>
const result = $('form').serializeJSON();
3. Convert the JavaScript object into a JSON string if needed.
const json = JSON.stringify(result);
4. Determine the data type (string by default) by using the :type
suffix or data-value-type
attribute:
<input type="text" name="excluded:skip" value="ignored field" /> <input type="text" name="numbers[1]:number" value="1" /> <input type="text" name="bools[true]:boolean" value="true" /> <input type="text" name="nulls[null]:null" value="null" /> <input type="text" name="arrays[list]:array" value="[1, 2, 3]"/> <input type="text" name="objects[dict]:object" value='{"key": "value"}' /> <input type="text" name="anarray" data-value-type="array" value="[1, 2, 3]"/>
5. Or specify a data type using the customTypes
function:
$('form').serializeJSON({ customTypes: { myType: (strVal, el) => { // strVal: is the input value as a string // el: is the dom element. $(el) would be the jQuery element }, } });
6. Handle the uncheck value of your checkbox:
<input type="checkbox" name="checked[b]:boolean" value="true" data-unchecked-value="false" checked /> <input type="checkbox" name="checked[numb]" value="1" data-unchecked-value="0" checked /> <input type="checkbox" name="checked[cool]" value="YUP" checked />
$('form').serializeJSON({ checkboxUncheckedValue: 'NOPE' });
7. Full configuration options:
$('form').serializeJSON({ // to include that value for unchecked checkboxes (instead of ignoring them) checkboxUncheckedValue: undefined, // name="foo[2]" value="v" => {foo: [null, null, "v"]}, instead of {foo: ["2": "v"]} useIntKeysAsArrayIndex: false, // skip serialization of falsy values for listed value types skipFalsyValuesForTypes: [], // skip serialization of falsy values for listed field names skipFalsyValuesForFields: [], // do not interpret ":type" suffix as a type disableColonTypes: false, // extends defaultTypes customTypes: {}, // default types defaultTypes: { "string": function(str) { return String(str); }, "number": function(str) { return Number(str); }, "boolean": function(str) { var falses = ["false", "null", "undefined", "", "0"]; return falses.indexOf(str) === -1; }, "null": function(str) { var falses = ["false", "null", "undefined", "", "0"]; return falses.indexOf(str) === -1 ? str : null; }, "array": function(str) { return JSON.parse(str); }, "object": function(str) { return JSON.parse(str); }, "skip": null // skip is a special type used to ignore fields }, // default type defaultType: "string", });
This awesome jQuery plugin is developed by marioizquierdo. For more Advanced Usages, please check the demo page or visit the official website.