Beautiful jQuery File Upload Plugin with Bootstrap
File Size: | 377 KB |
---|---|
Views Total: | 291065 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
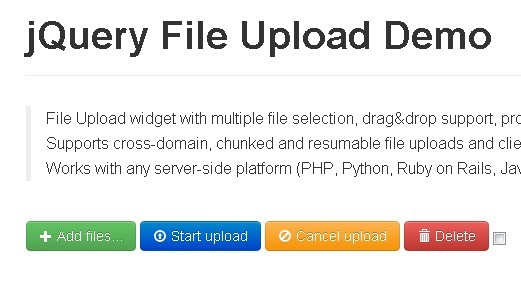
A Beautiful and powerful jQuery File Upload Plugin with support for multiple file selection, drag and drop, progress bar, image/audio/video preview, and much more.
Also supports cross-domain, file validation, chunked and resumable file uploads and client-side image resizing. This plugin is built with Twitter's Bootstrap toolkit and Icons from Glyphicon.
Note that the plugin requires a server-side upload handler to handle the file upload. Check out the files under the server folder for more information.
This is the 1st file upload plugin on jQueryScript.
Alternatives:
- Fine Uploader - User Friendly File Uploading Plugin
- Drag and Drop File Uploader Plugin - dropzone
- Elegant File Input Enhancement Plugin With JavaScript – filepond
Basic usage:
1. Load the JavaScript libraries in the HTML file.
<!-- Required --> <script src="/path/to/cdn/jquery.min.js"></script> <script src="js/vendor/jquery.ui.widget.js"></script> <script src="js/jquery.iframe-transport.js"></script> <script src="js/jquery.fileupload.js"></script> <!-- Audio Preview (OPTIONAL) --> <script src="js/jquery.fileupload-audio.js"></script> <!-- Image Preview & Resize (OPTIONAL) --> <script src="js/jquery.fileupload-image.js"></script> <!-- Upload Processing (OPTIONAL) --> <script src="js/jquery.fileupload-process.js"></script> <!-- User Interface (OPTIONAL) --> <script src="js/jquery.fileupload-ui.js"></script> <!-- File Validation (OPTIONAL) --> <script src="js/jquery.fileupload-validate.js"></script> <!-- Video Preview (OPTIONAL) --> <script src="js/jquery.fileupload-video.js"></script>
2. Create a file input and specify the path to the file handler.
<input id="fileupload" type="file" name="files[]" data-url="server/php/index.php" multiple>
3. Attach the plugin to the file input and specify the data type the server will return.
$(function () { $('#fileupload').fileupload({ dataType: 'json', done: function (e, data) { // do something here } }); });
4. Create a progress bar to visualize the upload progress.
<div id="progress"> <div class="bar" style="width: 0%;"></div> </div>
$(function () { $('#fileupload').fileupload({ dataType: 'json', done: function (e, data) { // do something here }, progressall: function (e, data) { var progress = parseInt(data.loaded / data.total * 100, 10); $('#progress .bar').css( 'width', progress + '%' ); } }); });
5. Possible options. The fileupload widget listens for change events on file input fields defined via fileInput setting and paste or drop events of the given dropZone. In addition to the default jQuery Widget methods, the fileupload widget exposes the "add" and "send" methods, to add or directly send files using the fileupload API. By default, files added via file input selection, paste, drag & drop or "add" method are uploaded immediately, but it is possible to override the "add" callback option to queue file uploads.
$('#fileupload').fileupload({ // The drop target element(s), by the default the complete document. // Set to null to disable drag & drop support: dropZone: $(document), // The paste target element(s), by the default undefined. // Set to a DOM node or jQuery object to enable file pasting: pasteZone: undefined, // The file input field(s), that are listened to for change events. // If undefined, it is set to the file input fields inside // of the widget element on plugin initialization. // Set to null to disable the change listener. fileInput: undefined, // By default, the file input field is replaced with a clone after // each input field change event. This is required for iframe transport // queues and allows change events to be fired for the same file // selection, but can be disabled by setting the following option to false: replaceFileInput: true, // The parameter name for the file form data (the request argument name). // If undefined or empty, the name property of the file input field is // used, or "files[]" if the file input name property is also empty, // can be a string or an array of strings: paramName: undefined, // By default, each file of a selection is uploaded using an individual // request for XHR type uploads. Set to false to upload file // selections in one request each: singleFileUploads: true, // To limit the number of files uploaded with one XHR request, // set the following option to an integer greater than 0: limitMultiFileUploads: undefined, // The following option limits the number of files uploaded with one // XHR request to keep the request size under or equal to the defined // limit in bytes: limitMultiFileUploadSize: undefined, // Multipart file uploads add a number of bytes to each uploaded file, // therefore the following option adds an overhead for each file used // in the limitMultiFileUploadSize configuration: limitMultiFileUploadSizeOverhead: 512, // Set the following option to true to issue all file upload requests // in a sequential order: sequentialUploads: false, // To limit the number of concurrent uploads, // set the following option to an integer greater than 0: limitConcurrentUploads: undefined, // Set the following option to true to force iframe transport uploads: forceIframeTransport: false, // Set the following option to the location of a redirect url on the // origin server, for cross-domain iframe transport uploads: redirect: undefined, // The parameter name for the redirect url, sent as part of the form // data and set to 'redirect' if this option is empty: redirectParamName: undefined, // Set the following option to the location of a postMessage window, // to enable postMessage transport uploads: postMessage: undefined, // By default, XHR file uploads are sent as multipart/form-data. // The iframe transport is always using multipart/form-data. // Set to false to enable non-multipart XHR uploads: multipart: true, // To upload large files in smaller chunks, set the following option // to a preferred maximum chunk size. If set to 0, null or undefined, // or the browser does not support the required Blob API, files will // be uploaded as a whole. maxChunkSize: undefined, // When a non-multipart upload or a chunked multipart upload has been // aborted, this option can be used to resume the upload by setting // it to the size of the already uploaded bytes. This option is most // useful when modifying the options object inside of the "add" or // "send" callbacks, as the options are cloned for each file upload. uploadedBytes: undefined, // By default, failed (abort or error) file uploads are removed from the // global progress calculation. Set the following option to false to // prevent recalculating the global progress data: recalculateProgress: true, // Interval in milliseconds to calculate and trigger progress events: progressInterval: 100, // Interval in milliseconds to calculate progress bitrate: bitrateInterval: 500, // By default, uploads are started automatically when adding files: autoUpload: true, // By default, duplicate file names are expected to be handled on // the server-side. If this is not possible (e.g. when uploading // files directly to Amazon S3), the following option can be set to // an empty object or an object mapping existing filenames, e.g.: // { "image.jpg": true, "image (1).jpg": true } // If it is set, all files will be uploaded with unique filenames, // adding increasing number suffixes if necessary, e.g.: // "image (2).jpg" uniqueFilenames: undefined, // Error and info messages: messages: { uploadedBytes: 'Uploaded bytes exceed file size' }, // Translation function, gets the message key to be translated // and an object with context specific data as arguments: i18n: function(message, context) { // eslint-disable-next-line no-param-reassign message = this.messages[message] || message.toString(); if (context) { $.each(context, function(key, value) { // eslint-disable-next-line no-param-reassign message = message.replace('{' + key + '}', value); }); } return message; }, // Additional form data to be sent along with the file uploads can be set // using this option, which accepts an array of objects with name and // value properties, a function returning such an array, a FormData // object (for XHR file uploads), or a simple object. // The form of the first fileInput is given as parameter to the function: formData: function(form) { return form.serializeArray(); }, // The add callback is invoked as soon as files are added to the fileupload // widget (via file input selection, drag & drop, paste or add API call). // If the singleFileUploads option is enabled, this callback will be // called once for each file in the selection for XHR file uploads, else // once for each file selection. // // The upload starts when the submit method is invoked on the data parameter. // The data object contains a files property holding the added files // and allows you to override plugin options as well as define ajax settings. // // Listeners for this callback can also be bound the following way: // .bind('fileuploadadd', func); // // data.submit() returns a Promise object and allows to attach additional // handlers using jQuery's Deferred callbacks: // data.submit().done(func).fail(func).always(func); add: function(e, data) { if (e.isDefaultPrevented()) { return false; } if ( data.autoUpload || (data.autoUpload !== false && $(this).fileupload('option', 'autoUpload')) ) { data.process().done(function() { data.submit(); }); } }, // Events. // You can also fire the events using .on(Event, function(){...}); // Callback for the submit event of each file upload: submit: function (e, data) {}, // Callback for the start of each file upload request: send: function (e, data) {}, // Callback for successful uploads: done: function (e, data) {}, // Callback for failed (abort or error) uploads: fail: function (e, data) {}, // Callback for completed (success, abort or error) requests: always: function (e, data) {}, // Callback for upload progress events: progress: function (e, data) {}, // Callback for global upload progress events: progressall: function (e, data) {}, // Callback for uploads start, equivalent to the global ajaxStart event: start: function (e) {}, // Callback for uploads stop, equivalent to the global ajaxStop event: stop: function (e) {}, // Callback for change events of the fileInput(s): change: function (e, data) {}, // Callback for paste events to the pasteZone(s): paste: function (e, data) {}, // Callback for drop events of the dropZone(s): drop: function (e, data) {}, // Callback for dragover events of the dropZone(s): dragover: function (e) {}, // Callback before the start of each chunk upload request (before form data initialization): chunkbeforesend: function (e, data) {}, // Callback for the start of each chunk upload request: chunksend: function (e, data) {}, // Callback for successful chunk uploads: chunkdone: function (e, data) {}, // Callback for failed (abort or error) chunk uploads: chunkfail: function (e, data) {}, // Callback for completed (success, abort or error) chunk upload requests: chunkalways: function (e, data) {}, // The plugin options are used as settings object for the ajax calls. // The following are jQuery ajax settings required for the file uploads: processData: false, contentType: false, cache: false, timeout: 0 });
Changelog:
v10.32.0 (2021-09-25)
- Updated dev dependencies.
v10.31.0 (2020-07-12)
- Use CSS media queries to define file name display.
v10.30.1 (2020-06-09)
- Override Exif data if browser auto-rotates.
v10.29.0 (2020-05-22)
- Update
v10.28.0 (2020-05-16)
- Update
v10.27.0 (2020-05-16)
- Update
v10.25.0 (2020-05-13)
- Update
v10.24.0 (2020-05-11)
- Update
v10.23.0 (2020-05-08)
- Update
v10.22.0 (2020-05-07)
- Update
v10.21.0 (2020-05-06)
- Update
v10.20.0 (2020-05-04)
- Update
v10.18.0 (2020-05-04)
- Update
v10.17.0 (2020-05-02)
- Define load-image-orientation as dependency.
v10.16.0 (2020-05-01)
- Update
v10.15.0 (2020-04-30)
- Update optional blueimp-load-image dependency.
v10.14.0 (2020-04-29)
- Compatibility with jQuery 3.5.0
v10.13.1 (2020-04-19)
- Fix Iframe Transport form submit for Safari 13.
v10.13.0 (2020-04-12)
- Update meta data parsing options.
v10.12.0 (2020-04-10)
- Save updated Exif Orientation data in image file.
v10.11.0 (2020-04-09)
- Support automatic browser image orientation.
v10.10.0 (2020-04-03)
- Update
v10.9.0 (2020-03-27)
- Update
v10.8.0 (2020-02-21)
- Update
v10.7.0 (2019-12-31)
- Update
v10.6.0 (2019-12-22)
- Update
v10.5.0 (2019-12-18)
- Update
v10.4.0 (2019-11-24)
- Update
v10.3.0 (2019-08-28)
- Update
v10.2.0 (2019-08-28)
- Update
v10.1.0 (2019-08-03)
- Update
v10.0.0 (2019-07-21)
- Update
v9.34.0 (2019-07-20)
- Update
v9.32.0 (2019-06-23)
- Update
v9.31.0 (2019-05-24)
- Update
v9.30.0 (2019-04-20)
- Use window.innerWidth to detect mobile devices.
v9.29.0 (2019-04-16)
- Hide file names on mobile devices.
v9.28.0 (2018-11-13)
- security update
v9.27.0 (2018-11-07)
- security update
v9.26.0 (2018-11-02)
- security update
v9.25.1 (2018-10-26)
- security update
v9.23.0 (2018-10-15)
- Update
v9.22.0 (2018-06-19)
- Update
v9.21.0 (2018-02-19)
- Update
v9.20.0 (2018-01-23)
- Automatically infer the url (and poster for YT) from the YouTube/Vimeo video ID.
v9.19.3 (2018-01-15)
- Update
v9.18.0 (2017-03-29)
- Update
v9.17.0 (2017-02-18)
- Update
v9.16.0 (2017-02-10)
- Update
v9.15.0 (2017-02-06)
- Update
v9.14.2 (2016-12-20)
- Update
v9.14.1 (2016-11-21)
- Update
v9.12.3 (2016-04-13)
- Update
v9.11.2 (2015-08-29)
- Updated AngularJS, jQuery and jQueryUI.
v9.11.0 (2015-08-11)
- Updated AngularJS, jQuery and jQueryUI.
v9.10.4 (2015-07-08)
- Fixed race condition when cancelling the second file selection.
v9.10.3 (2015-07-07)
- update.
v9.10.1 (2015-06-12)
- update.
v9.9.4 (2015-05-20)
- Fixed broken data attributes handling for IE<9.
v9.9.3 (2015-01-05)
- Fixed broken data attributes handling for IE<9.
v9.9.2 (2015-01-05)
- Apache+PHP security patch release.
v9.9.1 (2015-01-05)
- update.
v9.9.0 (2015-01-01)
- update.
v9.8.1 (2014-09-16)
- jQuery cache memory leaks patch release.
v9.7.2 (2014-09-16)
- HTTPS demo + Load Image path fix release.
v9.7.1 (2014-09-03)
- IE10+ drag&drop patch release.
v9.7.0 (2014-07-22)
- Minor error message release version.
v9.6.0 (2014-07-16)
- Provide a reference to the cloned file input.
v9.5.6 (2014-02-13)
- Refactored last merge to fix HTML5 data-attributes handling.
v9.5.5 (2014-02-12)
- Bugfix release.
v9.5.4 (2014-01-28)
- Updated jQuery UI Widget Factory and fixed AngularJS widget options handling.
v9.5.3 (2014-01-23)
- bugs fixed.
v9.5.2 (2013-12-13)
- Iframe Transport bugfix release.
v9.5.0 (2013-12-02)
- Improved memory handling with image processing
v9.4.1 (2013-11-22)
- Use scope.evalAsync to make sure the digest cycle is triggered.
v9.3.0 (2013-11-15)
- Added options limitMultiFileUploadSize and limitMultiFileUploadSizeOverhead
v9.2.0 (2013-11-12)
- Render the upload template before processing the files.
- Updated dependencies.
v9.1.0 (2013-11-09)
- Added imageQuality, imageType and imageForceResize options.
v9.0.0 (2013-10-31)
- Render the upload template before processing the files. This allows to abort the processing queue via the user interface.
- Provide a server result in fail callbacks.
- Catch imagick image detection exceptions.
v8.9.0 (2013-10-12)
- Make sure templates with fade class are hidden before the transition is applied.
v8.8.7 (2013-10-09)
- Apply file upload style fixes to IE < 8.
- Don't use CSS imports.
v8.8.3 (2013-08-30)
- updated to the latest version
v8.7.1 (2013-08-05)
- Respect the prependFiles option in the done and fail callbacks. Fixed prependFiles is not always used
v8.7.0 (2013-08-02)
- just added an updated jQuery UI example implementation.
v8.6.0 (2013-07-10)
- Use camelCase for JSON properties.
v8.5.1 (2013-07-09)
- Clarify the importance of the "X-Content-Type-Options: nosniff" header.
- Added play-pause control for the Gallery.
- Disable file selection button if browser doesn't support file inputs.
This awesome jQuery plugin is developed by blueimp. For more Advanced Usages, please check the demo page or visit the official website.