Bootstrap Dropdown Select Enhancement Plugin With jQuery
File Size: | 364 KB |
---|---|
Views Total: | 25573 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
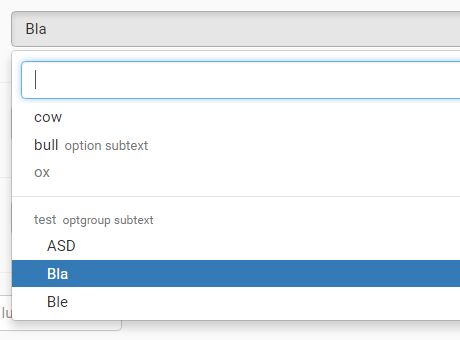
Bootstrap Select is a jQuery plugin for creating dropdown lists and menus using Bootstrap dropdown component that features live search, multiple selection, custom styling, select/deselect all and much more.
Bootstrap 4 Version is Now AVAILABLE.
Available extension:
Install the Bootstrap Select via Package managers:
# NPM npm install bootstrap-select # Bower bower install bootstrap-select
How to use it:
1. Import the Bootstrap Select plugin into your Bootstrap project. Note that the JavaScript file bootstrap-select.min.js
must be included after jQuery library.
<link rel="stylesheet" href="bootstrap-select.min.css"> <script src="bootstrap-select.min.js"></script>
2. Add the CSS class 'selectpicker' to your existing select
element as this:
<select id="basic" class="selectpicker form-control"> <option>cow</option> <option data-subtext="option subtext">bull</option> <option class="get-class" disabled>ox</option> <optgroup label="test" data-subtext="optgroup subtext"> <option>ASD</option> <option selected>Bla</option> <option>Ble</option> </optgroup> </select>
3. Call the function to initialize the plugin with default options.
$('.selectpicker').selectpicker({ // options here });
4. All possible plugin options to customize the dropdown select.
$('.selectpicker').selectpicker({ // text for no selection noneSelectedText: 'Nothing selected', // text for no result noneResultsText: 'No results matched {0}', // Sets the format for the text displayed when selectedTextFormat is count or count > #. {0} is the selected amount. {1} is total available for selection. // When set to a function, the first parameter is the number of selected options, and the second is the total number of options. // The function must return a string. countSelectedText: function (numSelected, numTotal) { return (numSelected == 1) ? "{0} item selected" : "{0} items selected"; }, // The text that is displayed when maxOptions is enabled and the maximum number of options for the given scenario have been selected. // If a function is used, it must return an array. array[0] is the text used when maxOptions is applied to the entire select element. array[1] is the text used when maxOptions is used on an optgroup. // If a string is used, the same text is used for both the element and the optgroup. maxOptionsText: function (numAll, numGroup) { return [ (numAll == 1) ? 'Limit reached ({n} item max)' : 'Limit reached ({n} items max)', (numGroup == 1) ? 'Group limit reached ({n} item max)' : 'Group limit reached ({n} items max)' ]; }, // Text for Select All button selectAllText: 'Select All', // Text for Deselect All button deselectAllText: 'Deselect All', // Shows done button doneButton: false, // Text for done button doneButtonText: 'Close', // custom separator multipleSeparator: ', ', // button styles styleBase: 'btn', style: 'btn-default', // dropdown size size: 'auto', // dropdown title title: null, // 'values' | 'static' | 'count' | 'count > x' selectedTextFormat: 'values', // dropdown width width: false, // e.g., container: 'body' | '.main-body' container: false, // hide disabled options hideDisabled: false, // shows sub text showSubtext: false, // shows icon showIcon: true, // shows content showContent: true, // auto dropup dropupAuto: true, // shows dropdown header header: false, // live search options liveSearch: false, liveSearchPlaceholder: null, liveSearchNormalize: false, liveSearchStyle: 'contains', // enables Select All / Deselect All box actionsBox: false, // icons iconBase: 'glyphicon', tickIcon: 'glyphicon-ok', // shows checkmark on selected option showTick: false, // custom template template: { caret: '<span class="caret"></span>' }, // string | array | function maxOptions: false, // enables the device's native menu for select menus mobile: false, // treats the tab character like the enter or space characters within the selectpicker dropdown. selectOnTab: false, // Align the menu to the right instead of the left. dropdownAlignRight: false, // e.g. [top, right, bottom, left] windowPadding: 0 });
5. Events available.
$('.selectpicker').on('show.bs.select', function (e) { // on show }); $('.selectpicker').on('shown.bs.select', function (e) { // on shown }); $('.selectpicker').on('hide.bs.select', function (e) { // on hide }); $('.selectpicker').on('hidden.bs.select', function (e) { // do hidden }); $('.selectpicker').on('loaded.bs.select', function (e) { // on loaded }); $('.selectpicker').on('rendered.bs.select', function (e) { // on rendered }); $('.selectpicker').on('refreshed.bs.select', function (e) { // on refreshed }); $('.selectpicker').on('changed.bs.select', function (e) { // on changed });
6. API methods.
// Sets the selected value $('.selectpicker').selectpicker('val', 'JQuery'); $('.selectpicker').selectpicker('val', ['jQuery','Script']); // Selects all items $('.selectpicker').selectpicker('selectAll'); // Clears all $('.selectpicker').selectpicker('deselectAll'); // Re-render $('.selectpicker').selectpicker('render'); // Enables mobile scrolling if( /Android|webOS|iPhone|iPad|iPod|BlackBerry/i.test(navigator.userAgent) ) { $('.selectpicker').selectpicker('mobile'); } // Sets styles // Replace Class $('.selectpicker').selectpicker('setStyle', 'btn-danger'); // Add Class $('.selectpicker').selectpicker('setStyle', 'btn-large', 'add'); // Remove Class $('.selectpicker').selectpicker('setStyle', 'btn-large', 'remove'); // Refreshes $('.selectpicker').selectpicker('refresh'); // Toggles the drop down list $('.selectpicker').selectpicker('toggle'); // Hides the drop down list $('.selectpicker').selectpicker('hide'); // Shows the drop down list $('.selectpicker').selectpicker('show'); // Destroys the drop down list $('.selectpicker').selectpicker('destroy');
Changelog:
v1.12.4 (2018-08-20)
- Bugfixed: Event creation throws illegal constructor error on stock Android Browser < 5.0
- Bugfixed: Bootstrap-select steals focus on form.checkValidity
v1.12.3 (2017-07-07)
- Add selectAllText and deselectAllText to translation files (used Google Translate)
- Keydown improvements
- Fixed htmlEscape inline style
- Livesearch performance
- Add/update various translations
This awesome jQuery plugin is developed by silviomoreto. For more Advanced Usages, please check the demo page or visit the official website.