Deselect Radio Buttons With jQuery
File Size: | 3.36 KB |
---|---|
Views Total: | 2066 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
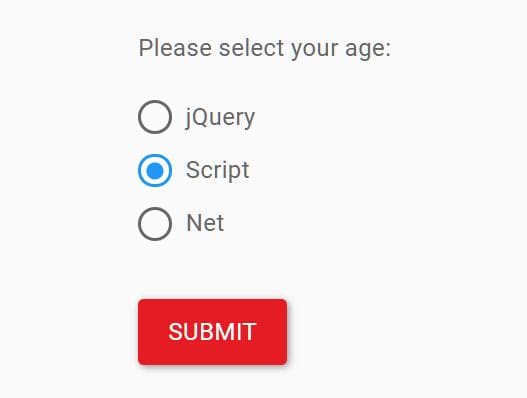
Most browser does not allow users to uncheck radio buttons by default. This may make users feel frustrated if they select a radio button option by mistake.
Here is a super tiny jQuery plugin (jQuery script) you might find useful that detects mouse/gesture events on radio buttons and allows the users to uncheck them with click or tap.
How to use it:
1. Load the necessary jQuery JavaScript library on the HTML page.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
2. Suppose you have a group of radio buttons on the page.
<input type="radio" id="age1" name="age" value="30"> <label for="age1">0 - 30</label><br> <input type="radio" id="age2" name="age" value="60"> <label for="age2">31 - 60</label><br> <input type="radio" id="age3" name="age" value="100"> <label for="age3">61 - 100</label>
3. The main JavaScript (jQuery script) to make these radio button unselectable just like checkboxes.
(function($){ $(document).ready(function(){ $(document.body).on('click mousedown keydown keyup', 'input[type="radio"]', function(e){ let radioButton = $(e.target); if(('keydown' === e.type && 32 === e.keyCode) || 'mousedown' === e.type){ radioButton.data('originally_checked', radioButton.prop('checked')); } if(('keyup' === e.type && 32 === e.keyCode) || 'click' === e.type){ if(radioButton.data('originally_checked')){ radioButton.prop('checked', false).removeAttr('checked'); } else { radioButton.prop('checked', true).attr('checked', 'checked'); } radioButton.removeData('originally_checked').trigger('done_changing'); } }); }); }(jQuery));
4. Or load the deselectable-radio-buttons.js
script after jQuery library. That's it.
<script src="deselectable-radio-buttons.js"></script>
This awesome jQuery plugin is developed by reedhewitt. For more Advanced Usages, please check the demo page or visit the official website.