Chunked File Uploader With jQury And PHP - fcup
File Size: | 11.9 KB |
---|---|
Views Total: | 5655 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
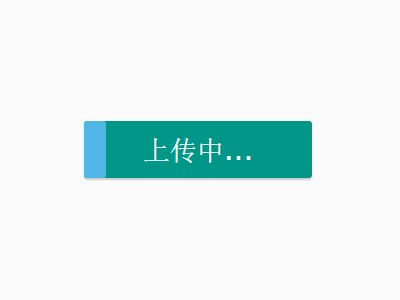
fcup is a simple, fully configurable Chunked File Uploader for larger files that supports both browser and node.js.
Features:
- Based on jQuery and PHP.
- Asynchronous upload.
- Uploads Chunked Files with an inline upload button.
- Inline Progress bar.
- Allows to specify the file types.
- Callback function.
Basic usage:
1. Create a container for the Chunked File Uploader.
<div class="fcup"></div>
2. Include jQuery JavaScript library and the fcup's JavaScript on the web page.
<script src="/path/to/jquery.min.js"></script> <script src="/path/to/jquery.fcup.min.js"></script>
3. Initialize the fcup and done.
$.fcup({ updom: '.fcup', upurl: 'file.php', // more options here });
4. Stylize the upload button in the CSS.
/* upload button */ .fcup-button { display: inline-block; font-size: 18px; color: #fff !important; text-decoration: none !important; padding: 10px 40px; line-height: 1; overflow: hidden; position: relative; box-shadow: 0 1px 1px #ccc; border-radius: 2px; background-color: #009688; background-image: -webkit-linear-gradient(top, #009688, #009688); background-image: -moz-linear-gradient(top, #009688, #009688); background-image: linear-gradient(top, #009688, #009688); } /* progress bar */ .fcup-button .tz-bar { background-color: #51b7e6; height: 3px; bottom: 0; left: 0; width: 0; position: absolute; z-index: 1; border-radius: 0 0 2px 2px; -webkit-transition: width 0.5s, height 0.5s; -moz-transition: width 0.5s, height 0.5s; transition: width 0.5s, height 0.5s; } .fcup-button.in-fcup, .fcup-button.finished { color: transparent !important; } .fcup-button.in-fcup:after, .fcup-button.finished:after { position: absolute; z-index: 2; width: 100%; height: 100%; text-align: center; top: 0; padding-top: inherit; color: #fff !important; left: 0; } .fcup-button.in-fcup:after { content: attr(data-loading); } .fcup-button.finished:after { content: attr(data-finished); } .fcup-button .tz-bar.background-horizontal { height: 100%; border-radius: 2px; } .fcup-button .tz-bar.background-vertical { height: 0; top: 0; width: 100%; border-radius: 2px; }
5. The example PHP to handle file uploading.
<?php /* header('Access-Control-Allow-Origin:*'); header('Access-Control-Allow-Methods:PUT,POST,GET,DELETE,OPTIONS'); header('Access-Control-Allow-Headers:x-requested-with,content-type'); */ header("Content-type: text/html; charset=utf-8"); $file = isset($_FILES['file_data']) ? $_FILES['file_data']:null; $name = isset($_POST['file_name']) ? '../upload/'.$_POST['file_name']:null; $total = isset($_POST['file_total']) ? $_POST['file_total']:0; $index = isset($_POST['file_index']) ? $_POST['file_index']:0; if(!$file || !$name){ echo 'failed'; die(); } if ($file['error'] == 0) { if (!file_exists($name)) { if (!move_uploaded_file($file['tmp_name'], $name)) { echo 'success'; } } else { $content = file_get_contents($file['tmp_name']); if (!file_put_contents($name, $content, FILE_APPEND)) { echo 'failed'; } echo 'success'; } } else { echo 'failed'; }
6. Possible options to customize the uploader.
$.fcup({ // selector for uploader updom: '.fcup', // 0.05mb shardsize : '0.05', // text for upload button upstr: 'Upload', // uploading text uploading: 'Uploading...', // text when finished upfinished: 'Finished', // PHP to handle file uploading upurl: './file.php', // allowed file types uptype: 'jpg,png,gif,jpeg,zip,rar', // text for invalid file type errtype: 'Invalid File Type' });
Change log:
2018-05-02
- added filesize valdation
- added MD5 support
- added more functions
- optimize codes.
2018-01-18
- Improvement and Fixing
This awesome jQuery plugin is developed by lovefc. For more Advanced Usages, please check the demo page or visit the official website.