Create A Pretty Contact Form With jQuery - Swyft_Callback
File Size: | 68.7 KB |
---|---|
Views Total: | 4988 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
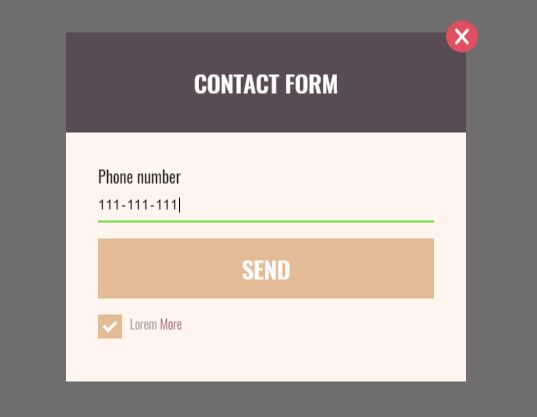
Swyft_Callback is a jQuery plugin used to create an animated, customizable, dynamic contact/feedback form with input mask integration in your web app.
How to use it:
1. Add the latest version of jQuery library and the jQuery Swyft_Callback plugin's files to the web page.
<script src="https://code.jquery.com/jquery-3.2.1.min.js" integrity="sha256-hwg4gsxgFZhOsEEamdOYGBf13FyQuiTwlAQgxVSNgt4=" crossorigin="anonymous"></script> <script src="swyft_callback.js"></script> <link rel="stylesheet" href="style.css">
2. Include the jQuery input mask plugin to create an input mask for your contact form.
<script src="jquery.inputmask.bundle.min.js"></script>
3. Call the function to create a basic toggle button on the web app that will toggle the contact form when clicked.
$( "body" ).swyftCallback();
4. Customize the contact form and toggle button.
$( "body" ).swyftCallback({ // api settings api: { url: 'test', custom: [ {name: 'api_key', value: ''}, ], param: { success: {name: 'result', value: 'success'}, //parameter named result will contain information about the call's success message: '', //the key of returned data (preferably an array) from the API which contains the response }, }, // data data: { form_method: "post", send_headers: true, custom_button_data: "", custom_popup_data: "", add_utm_params: false, utm_params_dictionary: ['utm_source', 'utm_medium', 'utm_campaign', 'utm_term', 'utm_content', 'keypartner'], }, // appearance appearance: { custom_button_class: "", custom_popup_class: "", show_check_all_agreements: true, overflown_overlay: true, ripple_effect: 1, //available: [1, 2], }, body_content: [], // status status: { popup_hidden: true, popup_body_collapsed: false, button_disabled: false, //disable show/close functionality ajax_processing: false, response_from_api_visible: true, }, // content - text text_vars: { popup_title: "Contact form", popup_body: "Leave us your phone number. We'll call you back.", send_button_text: "Send", wrong_input_text: "Wrong input", status_success: "Form sent successfuly", status_sending: "Sending form...", status_error: "Server encountered an error", }, // form info novalidate: true, // input settings input: { prefix: form_fields_prefix, fields: [], agreements: [], check_all_agreements: { obj: null, short: 'Check all agreements', }, regex_table: { inputmask: { phone: ["###-###-###", "## ###-##-##", "(###)###-####"] }, 'phone': /(\(?(\+|00)?48\)?([ -]?))?(\d{3}[ -]?\d{3}[ -]?\d{3})|([ -]?\d{2}[ -]?\d{3}[ -]?\d{2}[ -]?\d{2})/, 'email': /^(([^<>()[\]\\.,;:\s@\"]+(\.[^<>()[\]\\.,;:\s@\"]+)*)|(\".+\"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/, //^[a-zA-ZàáâäãåąčćęèéêëėįìíîïłńòóôöõøùúûüųūÿýżźñçčśšśžÀÁÂÄÃÅĄĆČĖĘÈÉÊËÌÍÎÏĮŁŃÒÓÔÖÕØÙÚÛÜŲŪŸÝŻŹÑßÇŒÆČŚŠŚŽ∂ð ,.'-]+$ 'name': /^[a-zA-Z\u00E0\u00E1\u00E2\u00E4\u00E3\u00E5\u0105\u010D\u0107\u0119\u00E8\u00E9\u00EA\u00EB\u0117\u012F\u00EC\u00ED\u00EE\u00EF\u0142\u0144\u00F2\u00F3\u00F4\u00F6\u00F5\u00F8\u00F9\u00FA\u00FB\u00FC\u0173\u016B\u00FF\u00FD\u017C\u017A\u00F1\u00E7\u010D\u015B\u0161\u015B\u017E\u00C0\u00C1\u00C2\u00C4\u00C3\u00C5\u0104\u0106\u010C\u0116\u0118\u00C8\u00C9\u00CA\u00CB\u00CC\u00CD\u00CE\u00CF\u012E\u0141\u0143\u00D2\u00D3\u00D4\u00D6\u00D5\u00D8\u00D9\u00DA\u00DB\u00DC\u0172\u016A\u0178\u00DD\u017B\u0179\u00D1\u00DF\u00C7\u0152\u00C6\u010C\u015A\u0160\u015A\u017D\u2202\u00F0 ,.'-]+$/, }, //dictionary is used to exchange input names into values from the dictionary on API request data_dictionary: {} //'sc_fld_telephone': 'phone' }, templates: { input: { field: { obj: null, name: 'phone', field_name: formObjPrefix + 'telephone', label: 'Phone number', type: 'tel', data_field_type: 'phone', //possible types: phone, name, email. Used for regex_table placeholder: '000-000-000', max_length: 20, required: true }, agreement: { obj: null, field_name: formObjPrefix + 'agreement', type: 'checkbox', short: 'Lorem', long: 'Ipsum', readmore: 'More', readless: 'Less', required: true, checked: true, }, }, body_content: { short: 'Short', long: 'Long', readmore: 'More', readless: 'Less', } }, // callbacks callbacks: { onShow: null, onHide: null, onSend: { success: { function: null, this: this, parameters: null, }, error: { function: null, this: this, parameters: null, } } } });
Changelog:
2018-10-04
- added inputmask support for email fields + fixed css for checkbox labels
2018-10-03
- bugfix check_all_agreements
2018-10-02
- no ripple by defeault
2018-09-12
- css - hide button text on mobile
2018-08-14
- update (cleanup references to jQuery elements)
2018-07-21
- input field, agreement and body_content template cleanup
2018-07-13
- css for form inputs update
2018-07-09
- fix css
2018-07-08
- fix reference issue on multiple plugin instances
2018-07-05
- fixed template
2018-07-03
- bugfix
2018-07-02
- bugfix
2018-06-19
- update inputmask
2018-06-11
- added string method calling
2018-05-19
- ES6 cleanup
2018-05-18
- optional add utm params option
2018-05-11
- Bugfix
2018-03-23
- local phone icons + css default icon appearance
2018-02-23
- cleanup js
2018-02-21
- fix js + wrong input status css
2018-01-29
- bugfix
2018-01-18
- fix input mask conflict
2018-01-13
- CSS fix
2017-12-15
- fix value for checkboxes in agreements
2017-12-13
- Callback return api result
2017-12-12
- custom callback on success/error from api
2017-12-07
- fix multiple fields validation bug
2017-12-06
- update swyft + responsiveness
2017-12-05
- Callback from ajax
- polishing + validation of fields and agreements
2017-12-04
- Added more customization options.
2017-12-01
- Bugfix
This awesome jQuery plugin is developed by Falkan3. For more Advanced Usages, please check the demo page or visit the official website.