Minimal Password Strength Indicator In jQuery
File Size: | 4.63 KB |
---|---|
Views Total: | 885 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
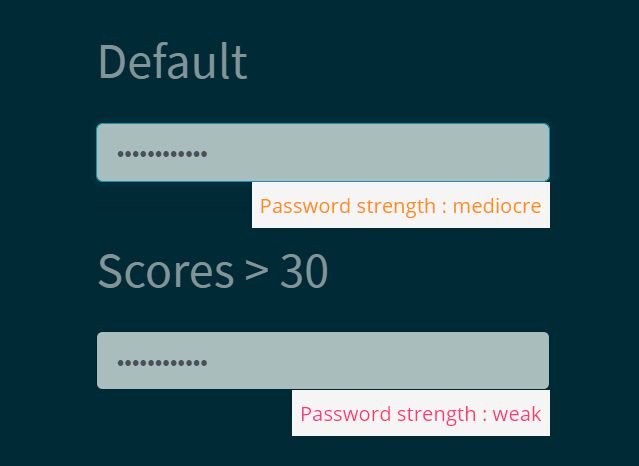
A minimal jQuery based password strength indicator that calculates the strength of a password based on certain criteria and displays the quality of the password in a visual feedback tooltip with custom messages.
The Default Strength Algorithm:
- For each Character: +1 point
- For each Space: +1 point
- Contains Lowercase Letter: +2 points
- Contains Uppercase Letter: +2 points
- Contains Number: +4 points
- Contains Special Character: +5 points
See Also:
How to use it:
1. Import the stylesheet jquery.password-indicator.css
and JavaScript jquery.password-indicator.js
into the HTML page.
<link rel="stylesheet" href="/path/to/jquery.password-indicator.css" /> <script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/jquery.password-indicator.js"></script>
2. Attach the Password Strength Indicator to a password input field you specify. That's it.
<input type="password" id="password" />
$(function() { $("#password").PasswordIndicator(); });
3. Override the default password strength algorithm:
$("#password").PasswordIndicator({ points: { forEachCharacter: 1, forEachSpace: 1, containsLowercaseLetter: 2, containsUppercaseLetter: 2, containsNumber: 4, containsSymbol: 5 } });
4. Determine the password strength you consider secure. Default: 25.
$("#password").PasswordIndicator({ secureStrength: 30 });
5. Customize the feedback messages & CSS classes:
$("#password").PasswordIndicator({ strengthClassNames: [{ name: "very-weak", text: "Password strength : very weak" }, { name: "weak", text: "Password strength : weak" }, { name: "mediocre", text: "Password strength : mediocre" }, { name: "strong", text: "Password strength : strong" }, { name: "awesome", text: "Password strength : Awesome" }] });
6. Customize the appearance of the password strength indicator.
$("#password").PasswordIndicator({ // place the indicator in another element $indicator: undefined, // CSS class indicatorClassName: "password-strength-indicator", // CSS DISPLAY property indicatorDisplayType: "inline-block", // CSS properties cssProperties: {"float": "right", "font-size": "13px"}, });
This awesome jQuery plugin is developed by harypurnomo. For more Advanced Usages, please check the demo page or visit the official website.