Powerful Form Validation Plugin For jQuery and Bootstrap 3
File Size: | 2 MB |
---|---|
Views Total: | 243285 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
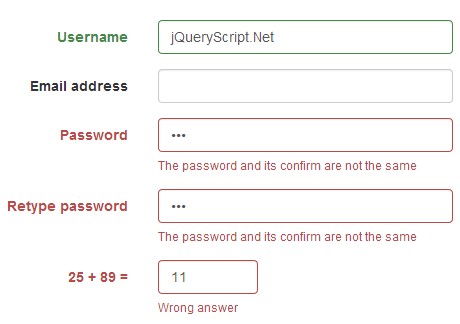
The plugin is NOT CURRENTLY Available For FREE DOWNLOAD. I recommend you to use the other form validation plugins instead.
Yet another jQuery and Bootstrap 3 powered form validation plugin to validate form values your user input with 16+ built-in validators. You can write new validators with Bootstrap Validator's APIs as well.
Available validators:
- between: Check if the input value is between (strictly or not) two given numbers
- callback: Return the validity from a callback method
- creditCard: alidate a credit card number
- different: Return true if the input value is different with given field's value
- digits: Return true if the value contains only digits
- emailAddress: Validate an email address
- greaterThan: Return true if the value is greater than or equals to given number
- hexColor: Validate a hex color
- identical: Check if the value is the same as one of particular field
- lessThan: Return true if the value is less than or equals to given number
- notEmpty: Check if the value is empty
- regexp: Check if the value matches given Javascript regular expression
- remote: Perform remote checking via Ajax request
- stringLength: Validate the length of a string
- uri: Validate an URL address
- usZipCode: Validate a US zip code
Basic Usage:
1. Include the required jQuery javascript library and Twitter's Bootstrap 3 in the page.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> <link rel="stylesheet" href="http://netdna.bootstrapcdn.com/bootstrap/3.0.2/css/bootstrap.min.css"> <script src="http://netdna.bootstrapcdn.com/bootstrap/3.0.2/js/bootstrap.min.js"></script>
2. Include jQuery Bootstrap Validator plugin after jQuery library.
<script type="text/javascript" src="../dist/js/bootstrapValidator.js"></script> <link rel="stylesheet" href="../dist/css/bootstrapValidator.css"/>
3. Create a sample sign in form with form validation functionality.
<form id="defaultForm" method="post" class="form-horizontal"> <div class="form-group"> <label class="col-lg-3 control-label">Username</label> <div class="col-lg-5"> <input type="text" class="form-control" name="username" /> </div> </div> <div class="form-group"> <label class="col-lg-3 control-label">Email address</label> <div class="col-lg-5"> <input type="text" class="form-control" name="email" /> </div> </div> <div class="form-group"> <label class="col-lg-3 control-label">Password</label> <div class="col-lg-5"> <input type="password" class="form-control" name="password" /> </div> </div> <div class="form-group"> <label class="col-lg-3 control-label">Retype password</label> <div class="col-lg-5"> <input type="password" class="form-control" name="confirmPassword" /> </div> </div> <div class="form-group"> <label class="col-lg-3 control-label" id="captchaOperation"></label> <div class="col-lg-2"> <input type="text" class="form-control" name="captcha" /> </div> </div> <div class="form-group"> <div class="col-lg-9 col-lg-offset-3"> <button type="submit" class="btn btn-primary">Sign up</button> </div> </div> </form>
4. The javascipt to apply the validators on the form and customize the warning messages when typing.
<script type="text/javascript"> $(document).ready(function() { // Generate a simple captcha function randomNumber(min, max) { return Math.floor(Math.random() * (max - min + 1) + min); }; $('#captchaOperation').html([randomNumber(1, 100), '+', randomNumber(1, 200), '='].join(' ')); $('#defaultForm').bootstrapValidator({ message: 'This value is not valid', fields: { username: { message: 'The username is not valid', validators: { notEmpty: { message: 'The username is required and can\'t be empty' }, stringLength: { min: 6, max: 30, message: 'The username must be more than 6 and less than 30 characters long' }, regexp: { regexp: /^[a-zA-Z0-9_\.]+$/, message: 'The username can only consist of alphabetical, number, dot and underscore' }, different: { field: 'password', message: 'The username and password can\'t be the same as each other' } } }, email: { validators: { notEmpty: { message: 'The email address is required and can\'t be empty' }, emailAddress: { message: 'The input is not a valid email address' } } }, password: { validators: { notEmpty: { message: 'The password is required and can\'t be empty' }, identical: { field: 'confirmPassword', message: 'The password and its confirm are not the same' }, different: { field: 'username', message: 'The password can\'t be the same as username' } } }, confirmPassword: { validators: { notEmpty: { message: 'The confirm password is required and can\'t be empty' }, identical: { field: 'password', message: 'The password and its confirm are not the same' }, different: { field: 'username', message: 'The password can\'t be the same as username' } } }, captcha: { validators: { callback: { message: 'Wrong answer', callback: function(value, validator) { var items = $('#captchaOperation').html().split(' '), sum = parseInt(items[0]) + parseInt(items[2]); return value == sum; } } } } } }); }); </script>
5. The default options.
// The first invalid field will be focused automatically autoFocus: true, // Support declarative usage (setting options via HTML 5 attributes) // Setting to false can improve the performance declarative: true, // The form CSS class elementClass: 'fv-form', // Use custom event name to avoid window.onerror being invoked by jQuery // See #630 events: { // Support backward formInit: 'init.form.fv', formError: 'err.form.fv', formSuccess: 'success.form.fv', fieldAdded: 'added.field.fv', fieldRemoved: 'removed.field.fv', fieldInit: 'init.field.fv', fieldError: 'err.field.fv', fieldSuccess: 'success.field.fv', fieldStatus: 'status.field.fv', localeChanged: 'changed.locale.fv', validatorError: 'err.validator.fv', validatorSuccess: 'success.validator.fv' }, // Indicate fields which won't be validated // By default, the plugin will not validate the following kind of fields: // - disabled // - hidden // - invisible // // The setting consists of jQuery filters. Accept 3 formats: // - A string. Use a comma to separate filter // - An array. Each element is a filter // - An array. Each element can be a callback function // function($field, validator) { // $field is jQuery object representing the field element // validator is the BootstrapValidator instance // return true or false; // } // // The 3 following settings are equivalent: // // 1) ':disabled, :hidden, :not(:visible)' // 2) [':disabled', ':hidden', ':not(:visible)'] // 3) [':disabled', ':hidden', function($field) { //return !$field.is(':visible'); // }] excluded: [':disabled', ':hidden', ':not(:visible)'], // Map the field name with validator rules fields: null, // Live validating option // Can be one of 3 values: // - enabled: The plugin validates fields as soon as they are changed // - disabled: Disable the live validating. The error messages are only shown after the form is submitted // - submitted: The live validating is enabled after the form is submitted live: 'enabled', // Locale in the format of languagecode_COUNTRYCODE locale: 'en_US', // Default invalid message message: 'This value is not valid', // The field will not be live validated if its length is less than this number of characters threshold: null, // Whether to be verbose when validating a field or not. // Possible values: // - true: when a field has multiple validators, all of them will be checked, and respectively - if errors occur in // multiple validators, all of them will be displayed to the user // - false: when a field has multiple validators, validation for this field will be terminated upon the first encountered error. // Thus, only the very first error message related to this field will be displayed to the user verbose: true, // ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ // These options mostly are overridden by specific framework // ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ button: { // The submit buttons selector // These buttons will be disabled to prevent the valid form from multiple submissions selector: '[type="submit"]', // The disabled class disabled: '' }, control: { // The CSS class for valid control valid: '', // The CSS class for invalid control invalid: '' }, err: { // The CSS class of each message element clazz: '', // The error messages container. It can be: // - 'tooltip' if you want to use Bootstrap tooltip to show error messages // - 'popover' if you want to use Bootstrap popover to show error messages // - a CSS selector indicating the container // In the first two cases, since the tooltip/popover should be small enough, the plugin only shows only one error message // You also can define the message container for particular field container: null, // Used to determine where the messages are placed parent: null }, // Shows ok/error/loading icons based on the field validity. icon: { valid: null, invalid: null, validating: null, feedback: '' }, row: { // The CSS selector for indicating the element consists of the field // You should adjust this option if your form group consists of many fields which not all of them need to be validated selector: null, valid: '', invalid: '', feedback: '' }
Change logs:
v0.6.1 (2015-02-24)
- Add dataType, crossDomain, validKey options for remote validator.
- Add declarative option to support big form
- Add Netherlands phone validator
- Add Bulgarian zip code validator
- Add Bulgarian phone number validator
- Add Polish zip code and id validators
- Support custom framework
- Remote validator fails if Ajax request fails
- Update Netherlands phone number validator
- Add plugin instance to the 3rd parameter of transformer callback
- Add Grunt task that runs the jasmine test suites
- Bug Fixes
v0.6.0 (2015-01-06)
- Add setLocale() and getLocale() methods to support multiple languages
- Add validateContainer() method
- Add transformer option, allowing to hook the value of field before validating
- Support add-on
- Add UAE phone number validator
- Add EIN validator
- Add BIC (ISO 9362) validator
- Add err, icon, row options
- Support Zurb Foundation framework
- Add Spanish postal code validator, thanks to @ethernet-zero
- Support Spanish CIF validator, thanks to @ethernet-zero
- Support UI Kit framework
- Support Pure framework
- Support Yahoo Pure framework
- Remove hexColor validator. Use color validator instead
- Showing only one message each time
- Add Required icon
- Google reCAPTCHA add-on
- multilingual add-on
- Add option to set optional protocol in uri validator
- Add clazz option
- Improve identical validator, thanks to @jazzzz
- Show the credit card icon based on its type
- Showing tooltip/popover when moving over or clicking the feedback icon (Bootstrap 3.3.0)
- Use jQuery instead of window.jQuery
- Allow to reuse data which is returned by the validator
- Don't need to set the different validator for both fields
- Improve the CPF validator
- Support Foundation framework
- Bugs fix.
- Document update.
- Rename to Form Validation
v0.5.3 (2014-11-05)
- Add min, max options for the date validator,
- Add color validator
- The stringLength validator adds option to evaluate length in UTF-8 bytes
- Add trim option for the stringLength validator
- Add minFiles, maxFiles, minTotalSize, maxTotalSize options for the file validator
- Add France postal code validator
- Add Ireland postal code validator
- Add German phone number and postal code validators
- Add Portugal postal code validator
- Add autoFocus option
- Add Austria and Switzerland postal code validators
- The hexColor validator only accepts 6 hex character values when using HTML 5 type='color' attribute
- Comma separator handling in greaterThan, lessThan validators
- Replace ',' with '.' to validate decimal numbers correct
- Put tooltip/popover on bottom if there is not enough space on top
- The remote validator allows to set data options via HTML attributes
- Enable validator when setting data-bv-validatorname="data-bv-validatorname"
- Requires jQuery 1.9.1 or higher
- Update the stringLength document
- Add a notification about setting identical validator for both fields
- Add Using language package example
- Update the Changing the tooltip, popover's position example
- Add time validator example
- Add Rails usage for stringLength validator
- Fix the order of parameters for enableFieldValidators() method
- Fix mixed data/delay in remote doc
- Updated docs for added German postal code and phone number validators
- Fix Changing tooltip, popover's position example link
- Languages update
v0.5.2 (2014-09-25)
- Add verbose option
- Add blank validator
- Add init and destroy methods to validator
- Add Venezuelan VAT number (RIF) validator
- Add China phone number validator
- Add Venezuela phone number validator
- Add Romania phone number validator
- Add Romania postal code validator
- Add Denmark phone number validator
- Add Thailand phone number and ID validator
- Add Chinese citizen ID validator
- Add Russia phone number validator
- Add Russian postal code validator
- Add Czech and Slovakia phone number and postal code validators
- Add delay option to the remote validator
- he different validator allows more than a 2-way comparison
- The container option can be defined by a callback
- Use CSS classes instead of inline styling to fix icons with input-group
- The stringLength validator supports HTML 5 minlength attribute
- The emailAddress validator accepts multiple email addresses
- Reuse data returned by callback, remote, custom validators
- The uri validator adds support for custom protocol
- Support VAT number without prefixing by country code
- Support latest Bootstrap when using tooltip/popover to show the message
- Improve behaviour of the different validator
- Add "BootstrapValidator's JavaScript requires jQuery" warning
- Add minSize option for the file validator
- Add phone number validator test suite
- Add Bootstrap Select and Select2 examples
- Add TinyMCE example
- Add Changing tooltip/popover position example
- Bugs fixed.
- Add more Language Packages.
v0.5.1 (2014-08-22)
- Add meid validator, thanks to @troymccabe
- Add imo validator, thanks to @troymccabe
- Add French phone number validator, thanks to @dlucazeau
- Add Spanish phone number validator, thanks to @vadail
- Add Iceland VAT number validator, thanks to @evilchili
- Add Pakistan phone number validator, thanks to @abuzer
- Add event name options to avoid window.onerror being invoked by jQuery, thanks to @roryprimrose.
- Add South African VAT number validator, thanks to @evilchili
- Add Brazilian phone number and postal code validator, thanks to @fhferreira
- Add zipCode and phone number validators for Morocco, thanks to @Arkni
- Add Brazilian VAT number validator, thanks to @fhferreira
v0.5.0 (2014-07-14)
- Major update.
- See CHANGELOG.md.
v0.4.5 (2014-05-15)
- Add $.fn.bootstrapValidator.helpers.date for validating a date, re-used in date, id, vat validators
- Add threshold option
- Add id validator
- Add separator option to the numeric validator
- Add isin (International Securities Identification Number) validator
- Add rtn (Routing transit number) validator
- Add cusip (North American Securities) validator
- Add sedol (Stock Exchange Daily Official List) validator
- The zipCode validator adds support for Italian, Dutch postcodes
- The cvv validator should support spaces in credit card, thanks to @evilchili
- Change default submitButtons to [type="submit"] to support input type="submit"
- Fix the conflict issue with MooTools
- The submit buttons are not sent
- The iban validator does not work on IE8
- Plugin method invocation don't work
- Fix the issue that the hidden fields generated by other plugins might not be validated
- When parsing options from HTML attributes, don't add the field which hasn't validators.
v0.4.5 (2014-05-07)
- Add threshold option
- When parsing options from HTML attributes, don't add the field which hasn't validators.
- Add id validator
v0.4.4 (2014-05-05)
- Add $.fn.bootstrapValidator.helpers.mod_11_10 method that implements modulus 11, 10 (ISO 7064) algorithm. The helper is then reused in validating German and Croatian VAT numbers
- Add $.fn.bootstrapValidator.helpers.mod_37_36 method that implements modulus 37, 36 (ISO 7064) algorithm, used in GRid validator
- Add EAN (International Article Number) validator
- Add GRId (Global Release Identifier) validator
- Add IMEI (International Mobile Station Equipment Identity) validator
- Add ISMN (International Standard Music Number) validator
- Add ISSN (International Standard Serial Number) validator
- Support using both the name attribute and selector option for field
- Indicate success/error tab
- Add UK postcode support for the zipCode validator
- The date validator supports seconds
- Wrong prefix of Laser credit card number
v0.4.3 (2014-04-26)
- Add file validator
- Add vat validator, support 32 countries
- Add Canadian Postal Code support for the zipCode validator, thanks to @Francismori7
- The choice validator supports select element
- Activate tab containing the first invalid field
- Plugin method invocation
- IE8 error
- The excluded: ':disabled' setting does not work on IE 8, thanks to @adgrafik
- The isbn validator accepts letters and special characters
v0.4.2 (2014-04-19)
- Add siren and siret validators
- Add Vehicle Identification Number (VIN) validator
- Add excluded option
- The phone validator now supports +1 country code and area code for US phone number
- The remote validator allows to override name option
- Do not validate fields that enabled is set to false
- Improve zipCode validator
v0.4.1 (2014-04-12)
- Fixed an issue that the custom submit handler is not fired from the second time
- Prevent the validate() method from submit the form automatically. So we can call validate() to validate the form
- Doesn't trigger validation on the first focus
- The row state is now only marked as success if all fields on it are valid
- Added support for element outside of form using the selector option
- User doesn't need to submit the form twice when remote validator complete
- Fix errors in IE 8
- The phone validator now also checks the length of US phone number
v0.4.0 (2014-04-03)
- Set validator option by using HTML 5 attributes (data-bv-*)
- Support HTML 5 input types
- Support multiple elements with the same name
- Add setLiveMode() method to turn on/off the live validating mode
- Add iban validator for validating IBAN (International Bank Account Number)
- Add uuid validator, support UUID v3, v4, v5
- Add numeric validator
- Add integer validator
- Add hex validator
- Add stringCase validator to check a string is lower or upper case
- Other improvements and fixes.
v0.3.3 (2014-03-27)
- FIXED: Don't validate disabled element
- FIXED: Cannot call form.submit() inside submitHandler
- FIXED: notEmpty validator doesn't work on file input
- FIXED: Handle case where a field is removed after the bootstrap validation
v0.3.2 (2014-03-21)
- Add selector option for each field. The field can be defined by CSS validator instead of the name attribute
- Add container option for each field to indicate where the error messages are shown
- Add ip validator. Support both IPv4 and IPv6
- Add isbn validator, support both ISBN 10 and ISBN 13
- Add step validator
- Add mac validator
- Add base64 validator
- Add cvv validator
- Add phone validator. Support US phone number only, thanks to @gercheq
v0.3.1 (2014-03-17)
- Add date validator
- Improve updateStatus() method to make the plugin play well with another
- Add enabled option and enableFieldValidators() method to enable/disable all validators to given field
- Add bower.json file, thanks to @ikanedo
- Support more form controls on the same row
- Remove the columns option. Now the plugin works normally no matter how many columns the form uses
- The resetForm method now only resets fields with validator rules
- FIXED: The error messages aren't shown if the form field doesn't have label
- FIXED: submitHandler or default submission isn't called after remote validation completes
2014-03-13
- Add date validator
- Add setNotValidated() method to make the plugin play well with another
- Add enabled option and enableFieldValidators() method to enable/disable all validators to given field
- Add bower.json file
- Support more form controls on the same row
- Remove the columns option
- Fixes: The error messages aren't shown if the form field doesn't have label
- Fixes: submitHandler or default submission isn't called after remote validation completes
2014-03-10
- Rewrite entirely using Deferred
- Add choice validator
- The remote validator supports dynamic data
- Add method to validate form manually
- Disable submit button on successful form submit
- Add submit button to submitHandler() parameter
- Add optional feedback icons
- Support Danish zip code
- Support Sweden zip code
- Support custom grid columns
- Show all errors
- Add resetForm() method
2014-03-08
- update
2014-02-17
- fixed validation for checkboxes
2014-02-14
- Fixed: Don't validate disabled element
- Fixed: Callback validator does not process if the field is empty
2014-02-07
- bugs fixed.
2014-01-23
- Fix the issue when the form label doesn't have class
v0.2.2 (2014-01-17)
- Focus to the first invalid element
- remote validator: Allow to set additional data to remote URL
- Avoid from calling form submit recursively
- Validate existing fields only
This awesome jQuery plugin is developed by formvalidation. For more Advanced Usages, please check the demo page or visit the official website.