Validate If A Password Meets Certain Requirements - PassRequirements
File Size: | 5.14 KB |
---|---|
Views Total: | 12398 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
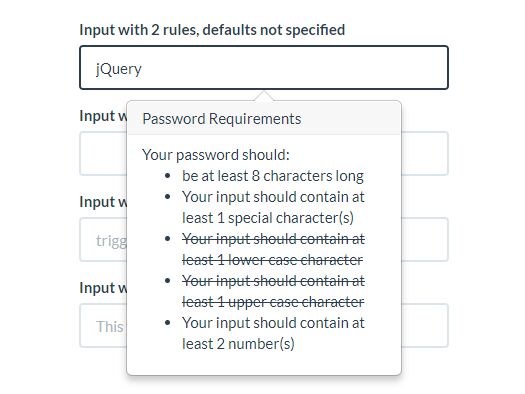
PassRequirements is a jQuery input field validation plugin that uses Bootstrap's tooltip component to provides an instant feedback when an input field (typically password field) meets certain requirements.
How use it:
1. The plugin requires jQuery library and Bootstrap framework loaded properly in the document.
<link rel="stylesheet" href="/path/to/bootstrap.min.css"> <script src="/path/to/jquery-3.2.1.slim.min.js"></script> <script src="/path/to/bootstrap.min.js"></script>
2. Load the main JavaScript file PassRequirements.js
after jQuery.
<script src="js/PassRequirements.js"></script>
3. Call the function on the input field and done. The default requirements:
- At least 8 characters long.
- At least 1 special character.
- At least 1 lower case character.
- At least 1 upper case character.
- At least 1 number.
<input id="test" class="form-control">
$('#test').PassRequirements();
4. Override or create your own validation rules in the JavaScript as follows:
$('#test').PassRequirements({ rules: { minlength: { text: "be at least minLength characters long", minLength: 8, }, containSpecialChars: { text: "Your input should contain at least minLength special character", minLength: 1, regex: new RegExp('([^!,%,&,@,#,$,^,*,?,_,~])', 'g') }, containLowercase: { text: "Your input should contain at least minLength lower case character", minLength: 1, regex: new RegExp('[^a-z]', 'g') }, containUppercase: { text: "Your input should contain at least minLength upper case character", minLength: 1, regex: new RegExp('[^A-Z]', 'g') }, containNumbers: { text: "Your input should contain at least minLength number", minLength: 1, regex: new RegExp('[^0-9]', 'g') } } });
This awesome jQuery plugin is developed by 09arnold. For more Advanced Usages, please check the demo page or visit the official website.