Dynamic Form Validator For Bootstrap - easyValidator
File Size: | 9.79 KB |
---|---|
Views Total: | 2310 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
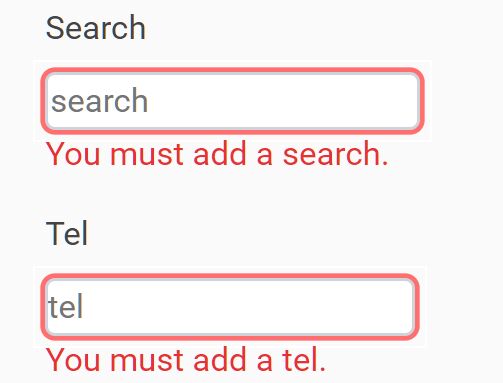
An easy jQuery form validation plugin for Bootstrap 4+ that dynamically applies custom validation rules to existing form controls using JavaScript.
Key features:
- Custom error messages.
- Allows to display error messages in the popover.
- Blinks the invalid form controls.
- Supports any form controls: input, select, checkbox, radio, etc.
- Do something when the form is valid/invalid.
How to use it:
1. Include the needed jQuery library and Bootstrap 4 framework on the page.
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous"> <script src="https://code.jquery.com/jquery-3.4.1.min.js" integrity="sha384-vk5WoKIaW/vJyUAd9n/wmopsmNhiy+L2Z+SBxGYnUkunIxVxAv/UtMOhba/xskxh" crossorigin="anonymous"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="sha384-UO2eT0CpHqdSJQ6hJty5KVphtPhzWj9WO1clHTMGa3JDZwrnQq4sF86dIHNDz0W1" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" integrity="sha384-JjSmVgyd0p3pXB1rRibZUAYoIIy6OrQ6VrjIEaFf/nJGzIxFDsf4x0xIM+B07jRM" crossorigin="anonymous"></script>
2. Download and include the easyValidator plugin's files on the page.
<link rel="stylesheet" href="easyValidator.min.css" /> <script src="easyValidator.min.js"></script>
3. Initialize the plugin and apply validation rules to existing form controls.
var isValid = $('.testform').easyValidator({ options: { style: 'blinked', // or 'normal' inputs: { email:{ type: 'input', reference: 'id', // identify your input referencevalue: 'inputEmail', msg: 'You must add an email.', showtype: 'p', // or 'popover' validateformat: true, validatemsg: 'The mail format is incorrect.' }, password: { type: 'input', reference: 'class', referencevalue: 'password', msg: 'You must add a password.', showtype: 'p' }, date: { type: 'input', reference: 'class', referencevalue: 'date', msg: 'You must add a date.', showtype: 'p' }, datetimelocal: { type: 'input', reference: 'class', referencevalue: 'datetime-local', msg: 'You must add a datetime-local.', showtype: 'p' }, file: { type: 'input', reference: 'class', referencevalue: 'file', msg: 'You must add a file.', showtype: 'p' }, month: { type: 'input', reference: 'class', referencevalue: 'month', msg: 'You must add a month.', showtype: 'popover', placement: "right" // placement }, range: { type: 'input', reference: 'class', referencevalue: 'range', msg: 'You must add a range.', showtype: 'p' }, search: { type: 'input', reference: 'class', referencevalue: 'search', msg: 'You must add a search.', showtype: 'p' }, tel: { type: 'input', reference: 'class', referencevalue: 'tel', msg: 'You must add a tel.', showtype: 'p' }, text: { type: 'input', reference: 'class', referencevalue: 'text', msg: 'You must add a text.', showtype: 'p' }, time: { type: 'input', reference: 'class', referencevalue: 'time', msg: 'You must add a time.', showtype: 'p' }, url: { type: 'input', reference: 'class', referencevalue: 'url', msg: 'You must add a url.', showtype: 'p' }, week: { type: 'input', reference: 'class', referencevalue: 'week', msg: 'You must add a week.', showtype: 'p' }, radios: { type: 'group', parenttype: 'id', parentvalue: 'radio-id', msg: 'You must select a radio button.', showtype: 'popover', placement: "right" }, checkbox: { type: 'group', parenttype: 'class', parentvalue: 'checkbox-group', msg: 'You must select a checkbox.', showtype: 'p' }, select:{ type: 'input', reference: 'id', referencevalue: 'gridSelect1', msg: 'You must select an element from the list.', showtype: 'p' }, textarea:{ type: 'input', reference: 'id', referencevalue: 'txtarea', msg: 'The textarea can not be null.', showtype: 'p' } } } });
4. Event handlers which will be fired when the form is valid or invalid.
if(isValid === true) { alert('Your isValid variable is equal to: '+ isValid + ', now you can proceed with your submit.'); } else { alert('Your isValid variable is equal to: '+ isValid +' , it seems there is an error left.'); }
This awesome jQuery plugin is developed by Mantixd. For more Advanced Usages, please check the demo page or visit the official website.