Amazon Like Drop Down Menu Plugin with jQuery - Menu Aim
File Size: | 1.58MB |
---|---|
Views Total: | 28464 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
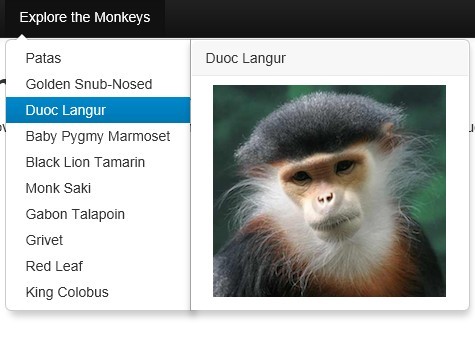
Menu Aim is a jQuery plugin inspired by Amazon's "Shop by Department" dropdown menu that can differentiate between a user trying hover over a dropdown item vs trying to navigate into a submenu's contents.
You might also like:
- Microsoft-Like Responsive Horizontal Drop Down Menu with CSS3 and jQuery
- Animated Drop Down Menu Plugin - apycom
- Pretty jQuery Navigation Menu With Smooth Animation Effect- tmailsilder
- Neat and modern Dropdown Nav Menu with Pure CSS3
- Responsive Multi-Level Navigation Plugin
- Pure CSS3 Drop Down Menu with Icons
- Smooth jQuery Dropdown Navigation Menu With CSS3
- Smooth and Responsive Drop Down Menu With CSS3 and jQuery
- Touch Enabled Multi-level Drop Down Menu Plugin - superfish
- Simple and Clean jQuery Drop Down Menu Plugin - naviDropDown
How to use it:
1. Include Twitter Bootstrap CSS files on your page
<link href="css/bootstrap.css" rel="stylesheet"> <link href="css/bootstrap-responsive.css" rel="stylesheet">
2. Markup html structure. Each class="popover" div defines submenu content. There are a million and one ways to do this, places to structure the HTML, etc. This is just one example. jQuery-menu-aim is agnostic to your HTML structure, it only fires events to be used as you please.
<div class="nav-collapse collapse"> <ul class="nav"> <li class="active"> <a class="dropdown-toggle" data-toggle="dropdown" href="#">Drop Down Menu</a> <ul class="dropdown-menu" role="menu"> <li data-submenu-id="submenu-menu1"> <a href="#">Menu1</a> <div id="submenu-patas" class="popover"> <h3 class="popover-title">Menu1 Title</h3> <div class="popover-content"><img src="img/menu1.png"></div> </div> </li> <li data-submenu-id="submenu-menu2"> <a href="#">Menu2</a> <div id="submenu-snub-nosed" class="popover"> <h3 class="popover-title">Menu2 Title</h3> <div class="popover-content"><img src="img/menu2.png"></div> </div> </li> ... </ul> </li> </ul> </div>
3. The CSS
<style> .popover { width: 400px; -webkit-border-top-left-radius: 0px; -webkit-border-bottom-left-radius: 0px; border-top-left-radius: 0px; border-bottom-left-radius: 0px; overflow: hidden; } .popover-content { text-align: center; } .popover-content img { height: 212px; max-width: 250px; } .dropdown-menu { -webkit-border-top-right-radius: 0px; -webkit-border-bottom-right-radius: 0px; border-top-right-radius: 0px; border-bottom-right-radius: 0px; -webkit-box-shadow: 5px 5px 10px rgba(0, 0, 0, 0.2); -moz-box-shadow: 5px 5px 10px rgba(0, 0, 0, 0.2); box-shadow: 5px 5px 10px rgba(0, 0, 0, 0.2); } .dropdown-menu > li > a:hover { background-image: none; color: white; background-color: rgb(0, 129, 194); background-color: rgba(0, 129, 194, 0.5); } .dropdown-menu > li > a.maintainHover { color: white; background-color: #0081C2; } </style>
4. Include jQuery library and other necessary javascript files on the pgae.
<script src="js/jquery-1.9.1.min.js"></script> <script src="../jquery.menu-aim.js"></script> <script src="js/bootstrap.min.js"></script>
5. The javascript
<script> var $menu = $(".dropdown-menu"); // jQuery-menu-aim: <meaningful part of the example> // Hook up events to be fired on menu row activation. $menu.menuAim({ activate: activateSubmenu, deactivate: deactivateSubmenu }); // jQuery-menu-aim: </meaningful part of the example> // jQuery-menu-aim: the following JS is used to show and hide the submenu // contents. Again, this can be done in any number of ways. jQuery-menu-aim // doesn't care how you do this, it just fires the activate and deactivate // events at the right times so you know when to show and hide your submenus. function activateSubmenu(row) { var $row = $(row), submenuId = $row.data("submenuId"), $submenu = $("#" + submenuId), height = $menu.outerHeight(), width = $menu.outerWidth(); // Show the submenu $submenu.css({ display: "block", top: -1, left: width - 3, // main should overlay submenu height: height - 4 // padding for main dropdown's arrow }); // Keep the currently activated row's highlighted look $row.find("a").addClass("maintainHover"); } function deactivateSubmenu(row) { var $row = $(row), submenuId = $row.data("submenuId"), $submenu = $("#" + submenuId); // Hide the submenu and remove the row's highlighted look $submenu.css("display", "none"); $row.find("a").removeClass("maintainHover"); } $(document).click(function() { // Simply hide the submenu on any click. Again, this is just a hacked // together menu/submenu structure to show the use of jQuery-menu-aim. $(".popover").css("display", "none"); $("a.maintainHover").removeClass("maintainHover"); }); </script>
This awesome jQuery plugin is developed by kamens. For more Advanced Usages, please check the demo page or visit the official website.